README.md
1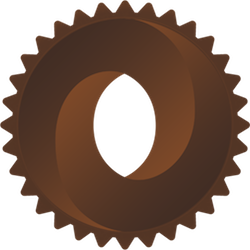
2
3# json-rust
4
5Parse and serialize [JSON](http://json.org/) with ease.
6
7**[Changelog](https://github.com/maciejhirsz/json-rust/releases) -**
8**[Complete Documentation](https://docs.rs/json/) -**
9**[Cargo](https://crates.io/crates/json) -**
10**[Repository](https://github.com/maciejhirsz/json-rust)**
11
12## Why?
13
14JSON is a very loose format where anything goes - arrays can hold mixed
15types, object keys can change types between API calls or not include
16some keys under some conditions. Mapping that to idiomatic Rust structs
17introduces friction.
18
19This crate intends to avoid that friction.
20
21```rust
22let parsed = json::parse(r#"
23
24{
25 "code": 200,
26 "success": true,
27 "payload": {
28 "features": [
29 "awesome",
30 "easyAPI",
31 "lowLearningCurve"
32 ]
33 }
34}
35
36"#).unwrap();
37
38let instantiated = object!{
39 // quotes on keys are optional
40 "code": 200,
41 success: true,
42 payload: {
43 features: [
44 "awesome",
45 "easyAPI",
46 "lowLearningCurve"
47 ]
48 }
49};
50
51assert_eq!(parsed, instantiated);
52```
53
54## First class citizen
55
56Using macros and indexing, it's easy to work with the data.
57
58```rust
59let mut data = object!{
60 foo: false,
61 bar: null,
62 answer: 42,
63 list: [null, "world", true]
64};
65
66// Partial equality is implemented for most raw types:
67assert!(data["foo"] == false);
68
69// And it's type aware, `null` and `false` are different values:
70assert!(data["bar"] != false);
71
72// But you can use any Rust number types:
73assert!(data["answer"] == 42);
74assert!(data["answer"] == 42.0);
75assert!(data["answer"] == 42isize);
76
77// Access nested structures, arrays and objects:
78assert!(data["list"][0].is_null());
79assert!(data["list"][1] == "world");
80assert!(data["list"][2] == true);
81
82// Error resilient - accessing properties that don't exist yield null:
83assert!(data["this"]["does"]["not"]["exist"].is_null());
84
85// Mutate by assigning:
86data["list"][0] = "Hello".into();
87
88// Use the `dump` method to serialize the data:
89assert_eq!(data.dump(), r#"{"foo":false,"bar":null,"answer":42,"list":["Hello","world",true]}"#);
90
91// Or pretty print it out:
92println!("{:#}", data);
93```
94
95## Installation
96
97Just add it to your `Cargo.toml` file:
98
99```toml
100[dependencies]
101json = "*"
102```
103
104Then import it in your `main.rs` / `lib.rs` file:
105
106```rust
107#[macro_use]
108extern crate json;
109```
110
111## Performance and Conformance
112
113There used to be a statement here saying that performance is not the main goal of this
114crate. It is definitely one of them now.
115
116While this crate doesn't provide a way to parse JSON to native Rust structs, it does a
117lot to optimize its performance for DOM parsing, stringifying and manipulation. It does
118[very well in benchmarks](https://github.com/serde-rs/json-benchmark), in some cases it
119can even outperform parsing to structs.
120
121This crate implements the standard according to the [
122RFC 7159](https://tools.ietf.org/html/rfc7159) and
123[ECMA-404](http://www.ecma-international.org/publications/files/ECMA-ST/ECMA-404.pdf)
124documents. For the best interoperability numbers are treated stored as 64bit precision
125mantissa with 16 bit decimal exponent for floating point representation.
126
127## License
128
129This crate is distributed under the terms of both the MIT license
130and the Apache License (Version 2.0). Choose whichever one works best for you.
131
132See [LICENSE-APACHE](LICENSE-APACHE) and [LICENSE-MIT](LICENSE-MIT) for details.
133