README.md
1itoa
2====
3
4[<img alt="github" src="https://img.shields.io/badge/github-dtolnay/itoa-8da0cb?style=for-the-badge&labelColor=555555&logo=github" height="20">](https://github.com/dtolnay/itoa)
5[<img alt="crates.io" src="https://img.shields.io/crates/v/itoa.svg?style=for-the-badge&color=fc8d62&logo=rust" height="20">](https://crates.io/crates/itoa)
6[<img alt="docs.rs" src="https://img.shields.io/badge/docs.rs-itoa-66c2a5?style=for-the-badge&labelColor=555555&logoColor=white&logo=data:image/svg+xml;base64,PHN2ZyByb2xlPSJpbWciIHhtbG5zPSJodHRwOi8vd3d3LnczLm9yZy8yMDAwL3N2ZyIgdmlld0JveD0iMCAwIDUxMiA1MTIiPjxwYXRoIGZpbGw9IiNmNWY1ZjUiIGQ9Ik00ODguNiAyNTAuMkwzOTIgMjE0VjEwNS41YzAtMTUtOS4zLTI4LjQtMjMuNC0zMy43bC0xMDAtMzcuNWMtOC4xLTMuMS0xNy4xLTMuMS0yNS4zIDBsLTEwMCAzNy41Yy0xNC4xIDUuMy0yMy40IDE4LjctMjMuNCAzMy43VjIxNGwtOTYuNiAzNi4yQzkuMyAyNTUuNSAwIDI2OC45IDAgMjgzLjlWMzk0YzAgMTMuNiA3LjcgMjYuMSAxOS45IDMyLjJsMTAwIDUwYzEwLjEgNS4xIDIyLjEgNS4xIDMyLjIgMGwxMDMuOS01MiAxMDMuOSA1MmMxMC4xIDUuMSAyMi4xIDUuMSAzMi4yIDBsMTAwLTUwYzEyLjItNi4xIDE5LjktMTguNiAxOS45LTMyLjJWMjgzLjljMC0xNS05LjMtMjguNC0yMy40LTMzLjd6TTM1OCAyMTQuOGwtODUgMzEuOXYtNjguMmw4NS0zN3Y3My4zek0xNTQgMTA0LjFsMTAyLTM4LjIgMTAyIDM4LjJ2LjZsLTEwMiA0MS40LTEwMi00MS40di0uNnptODQgMjkxLjFsLTg1IDQyLjV2LTc5LjFsODUtMzguOHY3NS40em0wLTExMmwtMTAyIDQxLjQtMTAyLTQxLjR2LS42bDEwMi0zOC4yIDEwMiAzOC4ydi42em0yNDAgMTEybC04NSA0Mi41di03OS4xbDg1LTM4Ljh2NzUuNHptMC0xMTJsLTEwMiA0MS40LTEwMi00MS40di0uNmwxMDItMzguMiAxMDIgMzguMnYuNnoiPjwvcGF0aD48L3N2Zz4K" height="20">](https://docs.rs/itoa)
7[<img alt="build status" src="https://img.shields.io/github/workflow/status/dtolnay/itoa/CI/master?style=for-the-badge" height="20">](https://github.com/dtolnay/itoa/actions?query=branch%3Amaster)
8
9This crate provides fast functions for printing integer primitives to an
10[`io::Write`] or a [`fmt::Write`]. The implementation comes straight from
11[libcore] but avoids the performance penalty of going through
12[`fmt::Formatter`].
13
14See also [`dtoa`] for printing floating point primitives.
15
16*Version requirement: rustc 1.0+*
17
18[`io::Write`]: https://doc.rust-lang.org/std/io/trait.Write.html
19[`fmt::Write`]: https://doc.rust-lang.org/core/fmt/trait.Write.html
20[libcore]: https://github.com/rust-lang/rust/blob/b8214dc6c6fc20d0a660fb5700dca9ebf51ebe89/src/libcore/fmt/num.rs#L201-L254
21[`fmt::Formatter`]: https://doc.rust-lang.org/std/fmt/struct.Formatter.html
22[`dtoa`]: https://github.com/dtolnay/dtoa
23
24```toml
25[dependencies]
26itoa = "0.4"
27```
28
29<br>
30
31## Performance (lower is better)
32
33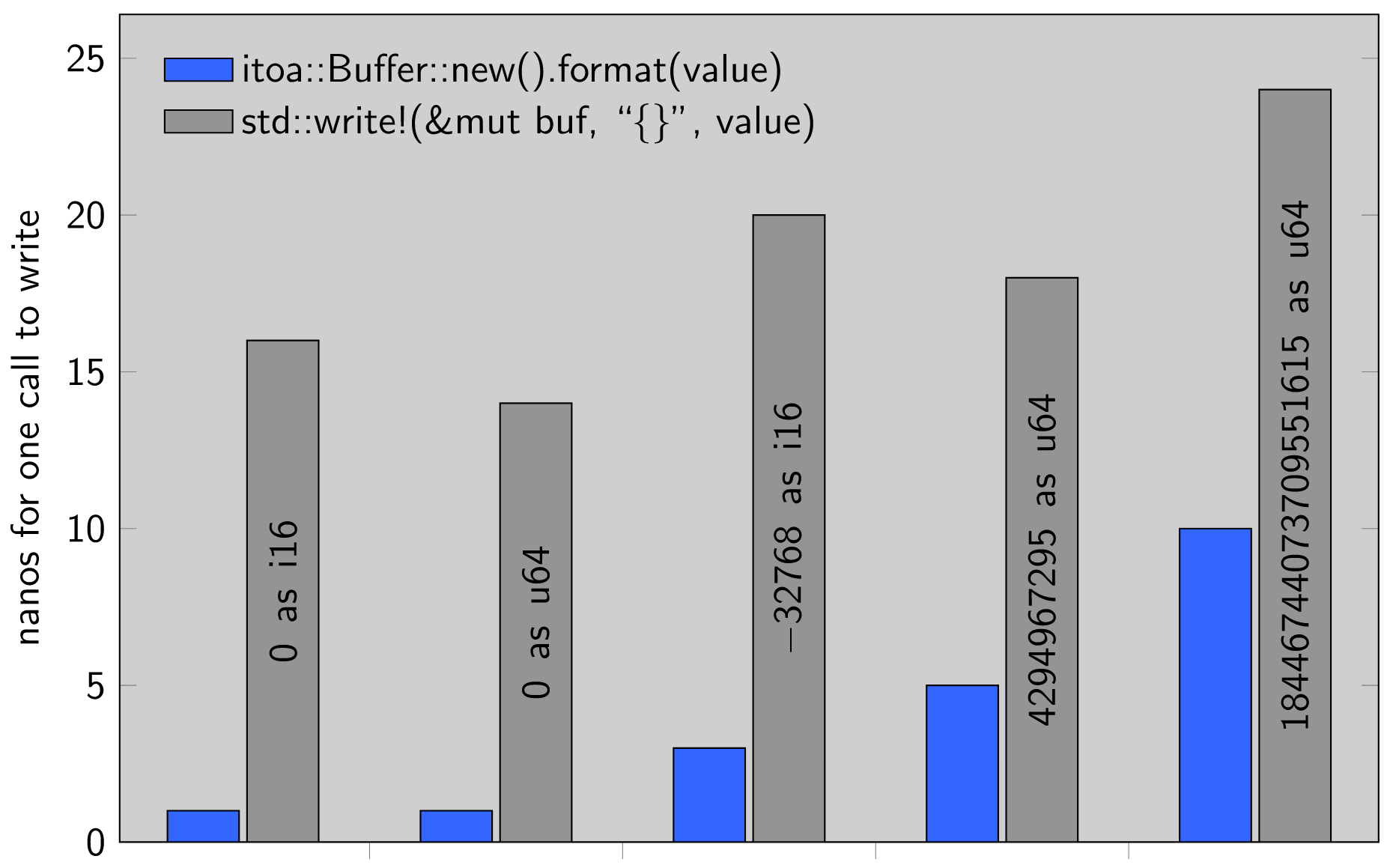
34
35<br>
36
37## Examples
38
39```rust
40use std::{fmt, io};
41
42fn demo_itoa_write() -> io::Result<()> {
43 // Write to a vector or other io::Write.
44 let mut buf = Vec::new();
45 itoa::write(&mut buf, 128u64)?;
46 println!("{:?}", buf);
47
48 // Write to a stack buffer.
49 let mut bytes = [0u8; 20];
50 let n = itoa::write(&mut bytes[..], 128u64)?;
51 println!("{:?}", &bytes[..n]);
52
53 Ok(())
54}
55
56fn demo_itoa_fmt() -> fmt::Result {
57 // Write to a string.
58 let mut s = String::new();
59 itoa::fmt(&mut s, 128u64)?;
60 println!("{}", s);
61
62 Ok(())
63}
64```
65
66The function signatures are:
67
68```rust
69fn write<W: io::Write, V: itoa::Integer>(writer: W, value: V) -> io::Result<usize>;
70
71fn fmt<W: fmt::Write, V: itoa::Integer>(writer: W, value: V) -> fmt::Result;
72```
73
74where `itoa::Integer` is implemented for i8, u8, i16, u16, i32, u32, i64, u64,
75i128, u128, isize and usize. 128-bit integer support requires rustc 1.26+ and
76the `i128` feature of this crate enabled.
77
78The `write` function is only available when the `std` feature is enabled
79(default is enabled). The return value gives the number of bytes written.
80
81<br>
82
83#### License
84
85<sup>
86Licensed under either of <a href="LICENSE-APACHE">Apache License, Version
872.0</a> or <a href="LICENSE-MIT">MIT license</a> at your option.
88</sup>
89
90<br>
91
92<sub>
93Unless you explicitly state otherwise, any contribution intentionally submitted
94for inclusion in this crate by you, as defined in the Apache-2.0 license, shall
95be dual licensed as above, without any additional terms or conditions.
96</sub>
97