README.md
1# react-plotly.js
2
3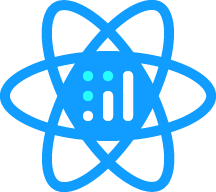
4
5> A [plotly.js](https://github.com/plotly/plotly.js) React component from
6> [Plotly](https://plot.ly/). The basis of Plotly's
7> [React component suite](https://plot.ly/products/react/).
8
9 [DEMO](http://react-plotly.js-demo.getforge.io/)
10
11 [Demo source code](https://github.com/plotly/react-plotly.js-demo-app)
12
13---
14
15## Contents
16
17- [Installation](#installation)
18- [Quick start](#quick-start)
19- [State management](#state-management)
20- [Refreshing the Plot](#refreshing-the-plot)
21- [API](#api)
22 - [Basic props](#basic-props)
23 - [Event handler props](#event-handler-props)
24- [Customizing the `plotly.js` bundle](#customizing-the-plotlyjs-bundle)
25- [Loading from a `<script>` tag](#loading-from-a-script-tag)
26- [Development](#development)
27
28## Installation
29
30```bash
31$ npm install react-plotly.js plotly.js
32```
33
34## Quick start
35
36The easiest way to use this component is to import and pass data to a plot component:
37
38```javascript
39import React from 'react';
40import Plot from 'react-plotly.js';
41
42class App extends React.Component {
43 render() {
44 return (
45 <Plot
46 data={[
47 {
48 x: [1, 2, 3],
49 y: [2, 6, 3],
50 type: 'scatter',
51 mode: 'lines+markers',
52 marker: {color: 'red'},
53 },
54 {type: 'bar', x: [1, 2, 3], y: [2, 5, 3]},
55 ]}
56 layout={{width: 320, height: 240, title: 'A Fancy Plot'}}
57 />
58 );
59 }
60}
61```
62
63You should see a plot like this:
64
65<p align="center">
66 <img src="example.png" alt="Example plot" width="320" height="240">
67</p>
68
69For a full description of Plotly chart types and attributes see the following resources:
70
71- [Plotly JavaScript API documentation](https://plot.ly/javascript/)
72- [Full plotly.js attribute listing](https://plot.ly/javascript/reference/)
73
74## State management
75
76This is a "dumb" component that doesn't merge its internal state with any updates. This means that if a user interacts with the plot, by zooming or panning for example, any subsequent re-renders will lose this information unless it is captured and upstreamed via the `onUpdate` callback prop.
77
78Here is a simple example of how to capture and store state in a parent object:
79
80```javascript
81class App extends React.Component {
82 constructor(props) {
83 super(props);
84 this.state = {data: [], layout: {}, frames: [], config: {}};
85 }
86
87 render() {
88 return (
89 <Plot
90 data={this.state.data}
91 layout={this.state.layout}
92 frames={this.state.frames}
93 config={this.state.config}
94 onInitialized={(figure) => this.setState(figure)}
95 onUpdate={(figure) => this.setState(figure)}
96 />
97 );
98 }
99}
100```
101
102## Refreshing the Plot
103
104This component will refresh the plot via [`Plotly.react`](https://plot.ly/javascript/plotlyjs-function-reference/#plotlyreact) if any of the following are true:
105
106- The `revision` prop is defined and has changed, OR;
107- One of `data`, `layout` or `config` has changed identity as checked via a shallow `===`, OR;
108- The number of elements in `frames` has changed
109
110Furthermore, when called, [`Plotly.react`](https://plot.ly/javascript/plotlyjs-function-reference/#plotlyreact) will only refresh the data being plotted if the _identity_ of the data arrays (e.g. `x`, `y`, `marker.color` etc) has changed, or if `layout.datarevision` has changed.
111
112In short, this means that simply adding data points to a trace in `data` or changing a value in `layout` will not cause a plot to update unless this is done immutably via something like [immutability-helper](https://github.com/kolodny/immutability-helper) if performance considerations permit it, or unless `revision` and/or [`layout.datarevision`](https://plot.ly/javascript/reference/#layout-datarevision) are used to force a rerender.
113
114## API Reference
115
116### Basic Props
117
118**Warning**: for the time being, this component may _mutate_ its `layout` and `data` props in response to user input, going against React rules. This behaviour will change in the near future once https://github.com/plotly/plotly.js/issues/2389 is completed.
119
120| Prop | Type | Default | Description |
121| ------------------ | ---------------------------- | ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
122| `data` | `Array` | `[]` | list of trace objects (see https://plot.ly/javascript/reference/) |
123| `layout` | `Object` | `undefined` | layout object (see https://plot.ly/javascript/reference/#layout) |
124| `frames` | `Array` | `undefined` | list of frame objects (see https://plot.ly/javascript/reference/) |
125| `config` | `Object` | `undefined` | config object (see https://plot.ly/javascript/configuration-options/) |
126| `revision` | `Number` | `undefined` | When provided, causes the plot to update when the revision is incremented. |
127| `onInitialized` | `Function(figure, graphDiv)` | `undefined` | Callback executed after plot is initialized. See below for parameter information. |
128| `onUpdate` | `Function(figure, graphDiv)` | `undefined` | Callback executed when a plot is updated due to new data or layout, or when user interacts with a plot. See below for parameter information. |
129| `onPurge` | `Function(figure, graphDiv)` | `undefined` | Callback executed when component unmounts, before `Plotly.purge` strips the `graphDiv` of all private attributes. See below for parameter information. |
130| `onError` | `Function(err)` | `undefined` | Callback executed when a plotly.js API method rejects |
131| `divId` | `string` | `undefined` | id assigned to the `<div>` into which the plot is rendered. |
132| `className` | `string` | `undefined` | applied to the `<div>` into which the plot is rendered |
133| `style` | `Object` | `{position: 'relative', display: 'inline-block'}` | used to style the `<div>` into which the plot is rendered |
134| `debug` | `Boolean` | `false` | Assign the graph div to `window.gd` for debugging |
135| `useResizeHandler` | `Boolean` | `false` | When true, adds a call to `Plotly.Plot.resize()` as a `window.resize` event handler |
136
137**Note**: To make a plot responsive, i.e. to fill its containing element and resize when the window is resized, use `style` or `className` to set the dimensions of the element (i.e. using `width: 100%; height: 100%` or some similar values) and set `useResizeHandler` to `true` while setting `layout.autosize` to `true` and leaving `layout.height` and `layout.width` undefined. This can be seen in action in [this CodePen](https://codepen.io/nicolaskruchten/pen/ERgBZX) and will implement the behaviour documented here: https://plot.ly/javascript/responsive-fluid-layout/
138
139#### Callback signature: `Function(figure, graphDiv)`
140
141The `onInitialized`, `onUpdate` and `onPurge` props are all functions which will be called with two arguments: `figure` and `graphDiv`.
142
143- `figure` is a serializable object with three keys corresponding to input props: `data`, `layout` and `frames`.
144 - As mentioned above, for the time being, this component may _mutate_ its `layout` and `data` props in response to user input, going against React rules. This behaviour will change in the near future once https://github.com/plotly/plotly.js/issues/2389 is completed.
145- `graphDiv` is a reference to the (unserializable) DOM node into which the figure was rendered.
146
147### Event handler props
148
149Event handlers for specific [`plotly.js` events](https://plot.ly/javascript/plotlyjs-events/) may be attached through the following props:
150
151| Prop | Type | Plotly Event |
152| ------------------------- | ---------- | ------------------------------ |
153| `onAfterExport` | `Function` | `plotly_afterexport` |
154| `onAfterPlot` | `Function` | `plotly_afterplot` |
155| `onAnimated` | `Function` | `plotly_animated` |
156| `onAnimatingFrame` | `Function` | `plotly_animatingframe` |
157| `onAnimationInterrupted` | `Function` | `plotly_animationinterrupted` |
158| `onAutoSize` | `Function` | `plotly_autosize` |
159| `onBeforeExport` | `Function` | `plotly_beforeexport` |
160| `onBeforeHover` | `Function` | `plotly_beforehover` |
161| `onButtonClicked` | `Function` | `plotly_buttonclicked` |
162| `onClick` | `Function` | `plotly_click` |
163| `onClickAnnotation` | `Function` | `plotly_clickannotation` |
164| `onDeselect` | `Function` | `plotly_deselect` |
165| `onDoubleClick` | `Function` | `plotly_doubleclick` |
166| `onFramework` | `Function` | `plotly_framework` |
167| `onHover` | `Function` | `plotly_hover` |
168| `onLegendClick` | `Function` | `plotly_legendclick` |
169| `onLegendDoubleClick` | `Function` | `plotly_legenddoubleclick` |
170| `onRelayout` | `Function` | `plotly_relayout` |
171| `onRelayouting` | `Function` | `plotly_relayouting` |
172| `onRestyle` | `Function` | `plotly_restyle` |
173| `onRedraw` | `Function` | `plotly_redraw` |
174| `onSelected` | `Function` | `plotly_selected` |
175| `onSelecting` | `Function` | `plotly_selecting` |
176| `onSliderChange` | `Function` | `plotly_sliderchange` |
177| `onSliderEnd` | `Function` | `plotly_sliderend` |
178| `onSliderStart` | `Function` | `plotly_sliderstart` |
179| `onSunburstClick` | `Function` | `plotly_sunburstclick` |
180| `onTransitioning` | `Function` | `plotly_transitioning` |
181| `onTransitionInterrupted` | `Function` | `plotly_transitioninterrupted` |
182| `onUnhover` | `Function` | `plotly_unhover` |
183
184## Customizing the `plotly.js` bundle
185
186By default, the `Plot` component exported by this library loads a precompiled version of all of `plotly.js`, so `plotly.js` must be installed as a peer dependency. This bundle is around 6Mb unminified, and minifies to just over 2Mb.
187
188If you do not wish to use this version of `plotly.js`, e.g. if you want to use a [different precompiled bundle](https://github.com/plotly/plotly.js/blob/master/dist/README.md#partial-bundles) or if your wish to [assemble you own customized bundle](https://github.com/plotly/plotly.js#modules), or if you wish to load `plotly.js` [from a CDN](https://github.com/plotly/plotly.js#use-the-plotlyjs-cdn-hosted-by-fastly), you can skip the installation of as a peer dependency (and ignore the resulting warning) and use the `createPlotComponent` method to get a `Plot` component, instead of importing it:
189
190```javascript
191// simplest method: uses precompiled complete bundle from `plotly.js`
192import Plot from 'react-plotly.js';
193
194// customizable method: use your own `Plotly` object
195import createPlotlyComponent from 'react-plotly.js/factory';
196const Plot = createPlotlyComponent(Plotly);
197```
198
199## Loading from a `<script>` tag
200
201For quick one-off demos on [CodePen](https://codepen.io/) or [JSFiddle](https://jsfiddle.net/), you may wish to just load the component directly as a script tag. We don't host the bundle directly, so you should never rely on this to work forever or in production, but you can use a third-party service to load the factory version of the component from, for example, [https://unpkg.com/react-plotly.js@latest/dist/create-plotly-component.js](https://unpkg.com/react-plotly.js@latest/dist/create-plotly-component.js).
202
203You can load plotly.js and the component factory with:
204
205```html
206<script src="https://cdn.plot.ly/plotly-latest.min.js"></script>
207<script src="https://unpkg.com/react-plotly.js@latest/dist/create-plotly-component.js"></script>
208```
209
210And instantiate the component with
211
212```javascript
213const Plot = createPlotlyComponent(Plotly);
214
215ReactDOM.render(
216 React.createElement(Plot, {
217 data: [{x: [1, 2, 3], y: [2, 1, 3]}],
218 }),
219 document.getElementById('root')
220);
221```
222
223You can see an example of this method in action
224[here](https://codepen.io/rsreusser/pen/qPgwwJ?editors=1010).
225
226## Development
227
228To get started:
229
230```bash
231$ npm install
232```
233
234To transpile from ES2015 + JSX into the ES5 npm-distributed version:
235
236```bash
237$ npm run prepublishOnly
238```
239
240To run the tests:
241
242```bash
243$ npm run test
244```
245
246## License
247
248© 2017-2020 Plotly, Inc. MIT License.
249