1<img src="https://cdn0.iconfinder.com/data/icons/material-design-ii-glyph/614/3010_-_Translate-512.png" width="100" align="left" /> 2 3# react-native-i18n 4 5Integrates [I18n.js](https://github.com/fnando/i18n-js) with React Native. Uses the user preferred locale as default. 6<br/> 7<br/> 8 9## Installation 10 11**Using yarn (recommended)** 12 13`$ yarn add react-native-i18n` 14 15**Using npm** 16 17`$ npm install react-native-i18n --save` 18 19## Automatic setup 20 21After installing the npm package you need to link the native modules. 22 23If you're using React-Native >= 0.29 just link the library with the command `react-native link`. 24 25If you're using React-Native < 0.29, install [rnpm](https://github.com/rnpm/rnpm) with the command `npm install -g rnpm` and then link the library with the command `rnpm link`. 26 27If you're having any issue you can also try to install the library manually as follows. 28 29## Automatic setup with Cocoapods 30 31After installing the npm package, add the following line to your Podfile 32 33```ruby 34pod 'RNI18n', :path => '../node_modules/react-native-i18n' 35``` 36 37and run 38 39``` 40pod install 41``` 42 43## Manual setup 44 45### iOS 46 47Add `RNI18n.xcodeproj` to **Libraries** and add `libRNI18n.a` to **Link Binary With Libraries** under **Build Phases**. 48[More info and screenshots about how to do this is available in the React Native documentation](http://facebook.github.io/react-native/docs/linking-libraries-ios.html#content). 49 50You also need to add the **localizations** you intend to support to your iOS project. To do that open your Xcode project: 51 52``` 53$ open <your-project>.xcodeproj 54``` 55 56And add the localizations you will support as shown here: 57 58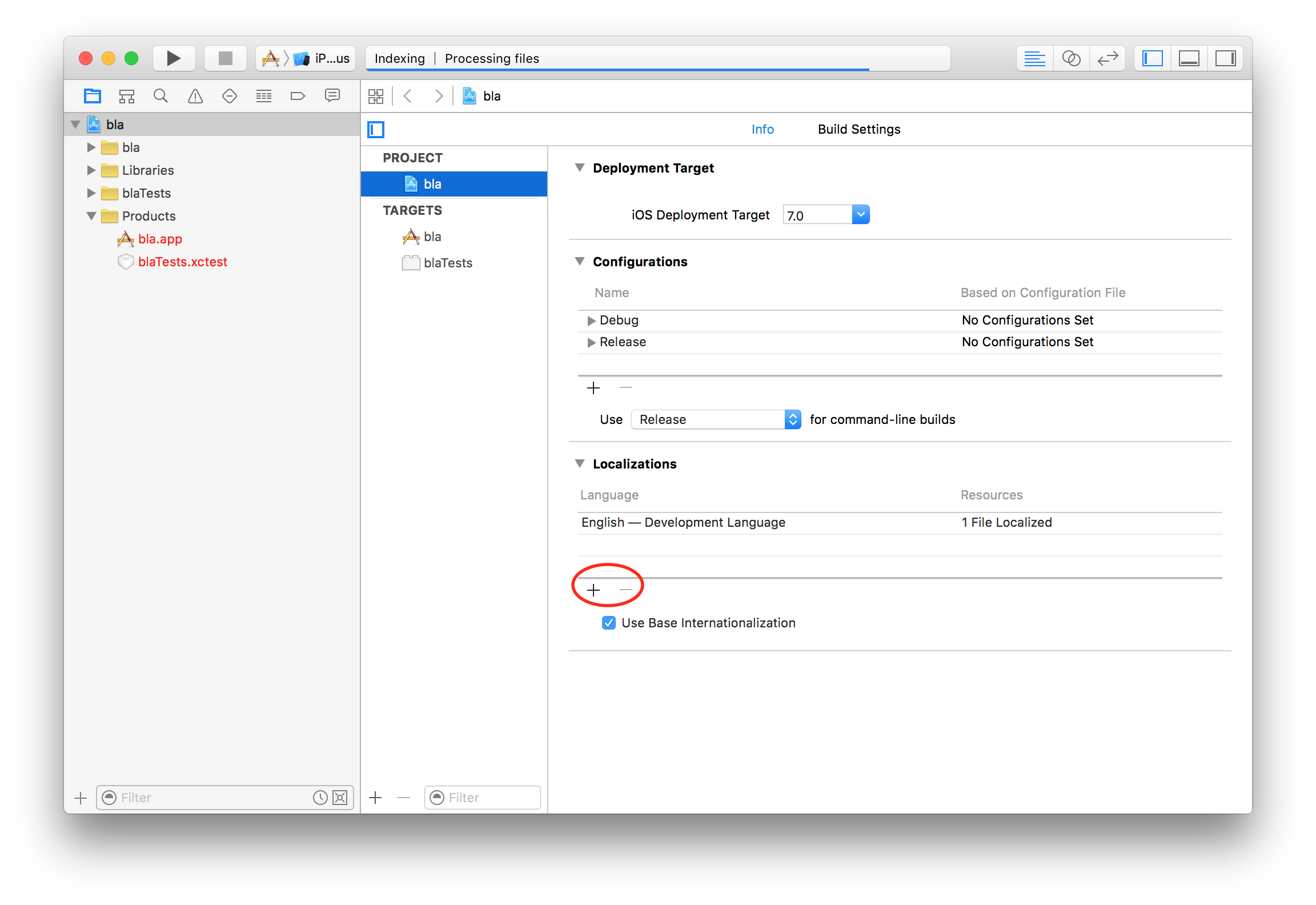 59 60### Android 61 62Add `react-native-i18n` to your `./android/settings.gradle` file as follows: 63 64```gradle 65include ':app', ':react-native-i18n' 66project(':react-native-i18n').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-i18n/android') 67``` 68 69Include it as dependency in `./android/app/build.gradle` file: 70 71```gradle 72dependencies { 73 // ... 74 compile project(':react-native-i18n') 75} 76``` 77 78Finally, you need to add the package to your MainApplication (`./android/app/src/main/java/your/bundle/MainApplication.java`): 79 80```java 81import com.AlexanderZaytsev.RNI18n.RNI18nPackage; // <-- Add to ReactNativeI18n to the imports 82 83// ... 84 85@Override 86protected List<ReactPackage> getPackages() { 87 return Arrays.<ReactPackage>asList( 88 new MainReactPackage(), 89 // ... 90 new RNI18nPackage(), // <-- Add it to the packages list 91 ); 92} 93 94// ... 95``` 96 97After that, you will need to recompile your project with `react-native run-android`. 98 99**⚠️ Important: You'll need to install Android build tools 27.0.3** 100 101## Usage 102 103```javascript 104import I18n from 'react-native-i18n'; 105// OR const I18n = require('react-native-i18n').default 106 107class Demo extends React.Component { 108 render() { 109 return <Text>{I18n.t('greeting')}</Text>; 110 } 111} 112 113// Enable fallbacks if you want `en-US` and `en-GB` to fallback to `en` 114I18n.fallbacks = true; 115 116I18n.translations = { 117 en: { 118 greeting: 'Hi!', 119 }, 120 fr: { 121 greeting: 'Bonjour!', 122 }, 123}; 124``` 125 126This will render `Hi!` for devices with the English locale, and `Bonjour!` for devices with the French locale. 127 128## Usage with multiple location files 129 130```javascript 131// app/i18n/locales/en.js 132 133export default { 134 greeting: 'Hi!' 135}; 136 137// app/i18n/locales/fr.js 138 139export default { 140 greeting: 'Bonjour!' 141}; 142 143// app/i18n/i18n.js 144 145import I18n from 'react-native-i18n'; 146import en from './locales/en'; 147import fr from './locales/fr'; 148 149I18n.fallbacks = true; 150 151I18n.translations = { 152 en, 153 fr 154}; 155 156export default I18n; 157 158// usage in component 159 160import I18n from 'app/i18n/i18n'; 161 162class Demo extends React.Component { 163 render () { 164 return ( 165 <Text>{I18n.t('greeting')}</Text> 166 ) 167 } 168} 169``` 170 171### Fallbacks 172 173When fallbacks are enabled (which is generally recommended), `i18n.js` will try to look up translations in the following order (for a device with `en_US` locale): 174 175- en-US 176- en 177 178**Note**: iOS 8 locales use underscored (`en_US`) but `i18n.js` locales are dasherized (`en-US`). This conversion is done automatically for you. 179 180```javascript 181I18n.fallbacks = true; 182 183I18n.translations = { 184 en: { 185 greeting: 'Hi!', 186 }, 187 'en-GB': { 188 greeting: 'Hi from the UK!', 189 }, 190}; 191``` 192 193For a device with a `en_GB` locale this will return `Hi from the UK!'`, for a device with a `en_US` locale it will return `Hi!`. 194 195### Device's locales 196 197You can get the user preferred locales with the `getLanguages` method: 198 199```javascript 200import { getLanguages } from 'react-native-i18n'; 201 202getLanguages().then(languages => { 203 console.log(languages); // ['en-US', 'en'] 204}); 205``` 206 207### I18n.js documentation 208 209For more info about I18n.js methods (`localize`, `pluralize`, etc) and settings see [its documentation](https://github.com/fnando/i18n-js#setting-up). 210 211## Licence 212 213MIT 214