README.md
1## Many-Core Engine for Perl
2
3This document describes MCE version 1.876.
4
5Many-Core Engine (MCE) for Perl helps enable a new level of performance by
6maximizing all available cores.
7
8
9
10### Description
11
12MCE spawns a pool of workers and therefore does not fork a new process per
13each element of data. Instead, MCE follows a bank queuing model. Imagine the
14line being the data and bank-tellers the parallel workers. MCE enhances that
15model by adding the ability to chunk the next n elements from the input
16stream to the next available worker.
17
18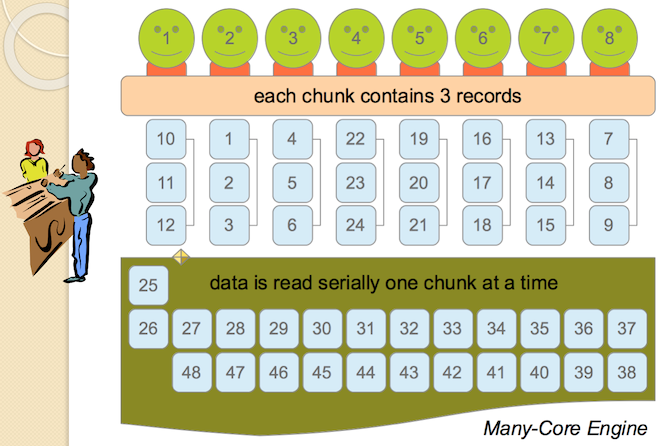
19
20### Synopsis
21
22This is a simplistic use case of MCE running with 5 workers.
23
24```perl
25 # Construction using the Core API
26
27 use MCE;
28
29 my $mce = MCE->new(
30 max_workers => 5,
31 user_func => sub {
32 my ($mce) = @_;
33 $mce->say("Hello from " . $mce->wid);
34 }
35 );
36
37 $mce->run;
38
39 # Construction using a MCE model
40
41 use MCE::Flow max_workers => 5;
42
43 mce_flow sub {
44 my ($mce) = @_;
45 MCE->say("Hello from " . MCE->wid);
46 };
47```
48
49The following is a demonstration for parsing a huge log file in parallel.
50
51```perl
52 use MCE::Loop;
53
54 MCE::Loop->init( max_workers => 8, use_slurpio => 1 );
55
56 my $pattern = 'something';
57 my $hugefile = 'very_huge.file';
58
59 my @result = mce_loop_f {
60 my ($mce, $slurp_ref, $chunk_id) = @_;
61
62 # Quickly determine if a match is found.
63 # Process the slurped chunk only if true.
64
65 if ($$slurp_ref =~ /$pattern/m) {
66 my @matches;
67
68 # The following is fast on Unix, but performance degrades
69 # drastically on Windows beyond 4 workers.
70
71 open my $MEM_FH, '<', $slurp_ref;
72 binmode $MEM_FH, ':raw';
73 while (<$MEM_FH>) { push @matches, $_ if (/$pattern/); }
74 close $MEM_FH;
75
76 # Therefore, use the following construction on Windows.
77
78 while ( $$slurp_ref =~ /([^\n]+\n)/mg ) {
79 my $line = $1; # save $1 to not lose the value
80 push @matches, $line if ($line =~ /$pattern/);
81 }
82
83 # Gather matched lines.
84
85 MCE->gather(@matches);
86 }
87
88 } $hugefile;
89
90 print join('', @result);
91```
92
93The next demonstration loops through a sequence of numbers with MCE::Flow.
94
95```perl
96 use MCE::Flow;
97
98 my $N = shift || 4_000_000;
99
100 sub compute_pi {
101 my ( $beg_seq, $end_seq ) = @_;
102 my ( $pi, $t ) = ( 0.0 );
103
104 foreach my $i ( $beg_seq .. $end_seq ) {
105 $t = ( $i + 0.5 ) / $N;
106 $pi += 4.0 / ( 1.0 + $t * $t );
107 }
108
109 MCE->gather( $pi );
110 }
111
112 # Compute bounds only, workers receive [ begin, end ] values
113
114 MCE::Flow->init(
115 chunk_size => 200_000,
116 max_workers => 8,
117 bounds_only => 1
118 );
119
120 my @ret = mce_flow_s sub {
121 compute_pi( $_->[0], $_->[1] );
122 }, 0, $N - 1;
123
124 my $pi = 0.0; $pi += $_ for @ret;
125
126 printf "pi = %0.13f\n", $pi / $N; # 3.1415926535898
127```
128
129### Installation and Dependencies
130
131To install this module type the following:
132
133 MCE_INSTALL_TOOLS=1 perl Makefile.PL (to include bin/mce_grep)
134 (or) perl Makefile.PL
135
136 make
137 make test
138 make install
139
140This module requires Perl 5.8.1 or later to run. By default, MCE spawns threads
141on Windows and child processes otherwise on Cygwin and Unix platforms. The use
142of threads requires that you include threads support prior to loading MCE.
143
144 processes (or) use threads; (or) use forks;
145 use threads::shared; use forks::shared;
146 use MCE; use MCE; use MCE;
147
148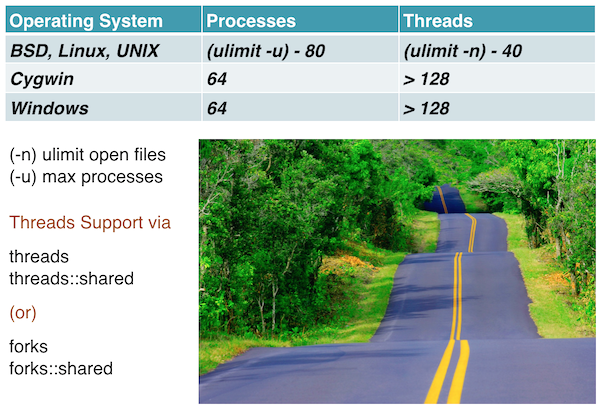
149
150MCE utilizes the following modules, which are mostly installed with Perl:
151
152 bytes
153 constant
154 Carp
155 Errno
156 Fcntl
157 File::Path
158 IO::Handle
159 Scalar::Util
160 Sereal::Decoder 3.015+ (optional)
161 Sereal::Encoder 3.015+ (optional)
162 Socket
163 Storable 2.04+ (default when Sereal isn't available)
164 Test::More 0.45+ (for make test only)
165 Time::HiRes
166
167### Further Reading
168
169The Perl MCE module is described at https://metacpan.org/pod/MCE.
170
171MCE options are described at [metacpan](https://metacpan.org/pod/MCE::Core).
172It includes several demonstrations at the end of the page.
173
174See [MCE::Examples](https://metacpan.org/pod/MCE::Examples)
175and [MCE Cookbook](https://github.com/marioroy/mce-cookbook)
176for more recipes.
177
178### Copyright and Licensing
179
180Copyright (C) 2012-2021 by Mario E. Roy <marioeroy AT gmail DOT com>
181
182This program is free software; you can redistribute it and/or modify
183it under the same terms as Perl itself:
184
185 a) the GNU General Public License as published by the Free
186 Software Foundation; either version 1, or (at your option) any
187 later version, or
188
189 b) the "Artistic License" which comes with this Kit.
190
191This program is distributed in the hope that it will be useful,
192but WITHOUT ANY WARRANTY; without even the implied warranty of
193MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See either
194the GNU General Public License or the Artistic License for more details.
195
196You should have received a copy of the Artistic License with this
197Kit, in the file named "LICENSE". If not, I'll be glad to provide one.
198
199You should also have received a copy of the GNU General Public License
200along with this program in the file named "Copying". If not, write to the
201Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor,
202Boston, MA 02110-1301, USA or visit their web page on the internet at
203http://www.gnu.org/copyleft/gpl.html.
204
205