1import pytest 2from django.template.loader import render_to_string 3 4from tests.thumbnail_tests.utils import BaseTestCase 5 6 7pytestmark = pytest.mark.django_db 8 9 10class FilterTestCase(BaseTestCase): 11 def test_html_filter(self): 12 text = '<img alt="A image!" src="http://dummyimage.com/800x800" />' 13 val = render_to_string('htmlfilter.html', {'text': text, }).strip() 14 15 self.assertEqual( 16 '<img alt="A image!" ' 17 'src="/media/test/cache/2e/35/2e3517d8aa949728b1ee8b26c5a7bbc4.jpg" />', 18 val 19 ) 20 21 def test_html_filter_local_url(self): 22 text = '<img alt="A image!" src="/media/500x500.jpg" />' 23 val = render_to_string('htmlfilter.html', {'text': text, }).strip() 24 25 self.assertEqual( 26 '<img alt="A image!" ' 27 'src="/media/test/cache/c7/f2/c7f2880b48e9f07d46a05472c22f0fde.jpg" />', 28 val 29 ) 30 31 def test_markdown_filter(self): 32 text = '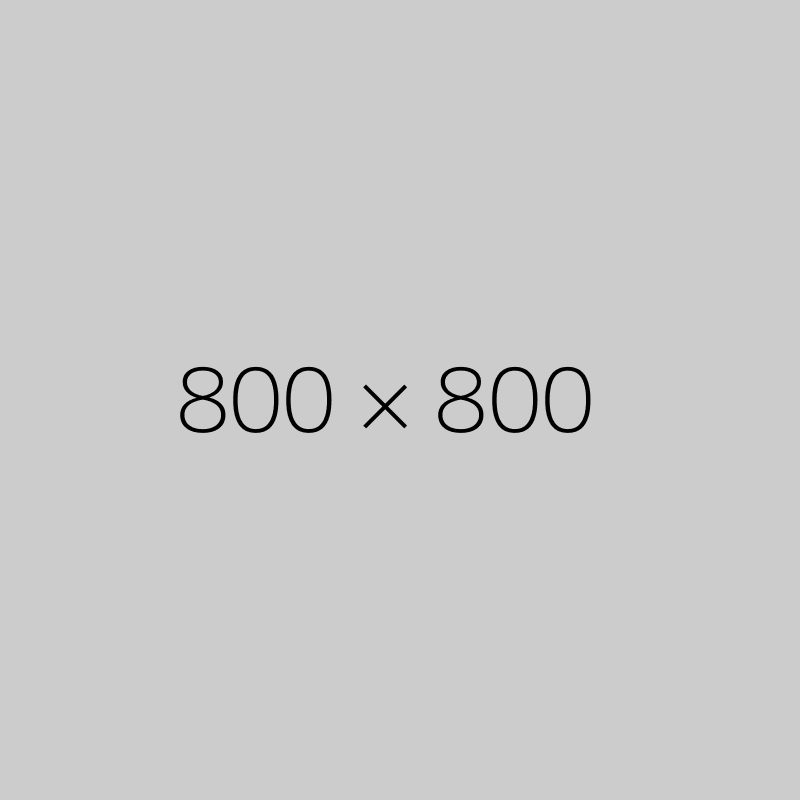' 33 val = render_to_string('markdownfilter.html', {'text': text, }).strip() 34 35 self.assertEqual( 36 '', 37 val 38 ) 39 40 def test_markdown_filter_local_url(self): 41 text = '' 42 val = render_to_string('markdownfilter.html', {'text': text, }).strip() 43 44 self.assertEqual( 45 '', 46 val 47 ) 48