1Metadata-Version: 2.1 2Name: textX 3Version: 2.3.0 4Summary: Meta-language for DSL implementation inspired by Xtext 5Home-page: https://github.com/textX/textX 6Author: Igor R. Dejanovic 7Author-email: igor.dejanovic@gmail.com 8License: MIT 9Description:  10 11 [](https://pypi.python.org/pypi/textX) 12  13 [](https://travis-ci.org/textX/textX) 14 [](https://coveralls.io/github/textX/textX?branch=master) 15 [](http://textx.github.io/textX/latest/) 16 17 18 textX is a meta-language for building Domain-Specific Languages (DSLs) in 19 Python. It is inspired by [Xtext]. 20 21 In a nutshell, textX will help you build your textual language in an easy way. 22 You can invent your own language or build a support for already existing textual 23 language or file format. 24 25 From a single language description (grammar), textX will build a parser and a 26 meta-model (a.k.a. abstract syntax) for the language. See the docs for the 27 details. 28 29 textX follows the syntax and semantics of Xtext but [differs in some 30 places](http://textx.github.io/textX/latest/about/comparison/) and is 31 implemented 100% in Python using [Arpeggio] PEG parser - no grammar ambiguities, 32 unlimited lookahead, interpreter style of work. 33 34 35 ## Quick intro 36 37 Here is a complete example that shows the definition of a simple DSL for 38 drawing. We also show how to define a custom class, interpret models and search 39 for instances of a particular type. 40 41 ```python 42 from textx import metamodel_from_str, get_children_of_type 43 44 grammar = """ 45 Model: commands*=DrawCommand; 46 DrawCommand: MoveCommand | ShapeCommand; 47 ShapeCommand: LineTo | Circle; 48 MoveCommand: MoveTo | MoveBy; 49 MoveTo: 'move' 'to' position=Point; 50 MoveBy: 'move' 'by' vector=Point; 51 Circle: 'circle' radius=INT; 52 LineTo: 'line' 'to' point=Point; 53 Point: x=INT ',' y=INT; 54 """ 55 56 # We will provide our class for Point. 57 # Classes for other rules will be dynamically generated. 58 class Point(object): 59 def __init__(self, parent, x, y): 60 self.parent = parent 61 self.x = x 62 self.y = y 63 64 def __str__(self): 65 return "{},{}".format(self.x, self.y) 66 67 def __add__(self, other): 68 return Point(self.parent, self.x + other.x, self.y + other.y) 69 70 # Create meta-model from the grammar. Provide `Point` class to be used for 71 # the rule `Point` from the grammar. 72 mm = metamodel_from_str(grammar, classes=[Point]) 73 74 model_str = """ 75 move to 5, 10 76 line to 10, 10 77 line to 20, 20 78 move by 5, -7 79 circle 10 80 line to 10, 10 81 """ 82 83 # Meta-model knows how to parse and instantiate models. 84 model = mm.model_from_str(model_str) 85 86 # At this point model is a plain Python object graph with instances of 87 # dynamically created classes and attributes following the grammar. 88 89 def cname(o): 90 return o.__class__.__name__ 91 92 # Let's interpret the model 93 position = Point(None, 0, 0) 94 for command in model.commands: 95 if cname(command) == 'MoveTo': 96 print('Moving to position', command.position) 97 position = command.position 98 elif cname(command) == 'MoveBy': 99 position = position + command.vector 100 print('Moving by', command.vector, 'to a new position', position) 101 elif cname(command) == 'Circle': 102 print('Drawing circle at', position, 'with radius', command.radius) 103 else: 104 print('Drawing line from', position, 'to', command.point) 105 position = command.point 106 print('End position is', position) 107 108 # Output: 109 # Moving to position 5,10 110 # Drawing line from 5,10 to 10,10 111 # Drawing line from 10,10 to 20,20 112 # Moving by 5,-7 to a new position 25,13 113 # Drawing circle at 25,13 with radius 10 114 # Drawing line from 25,13 to 10,10 115 116 # Collect all points starting from the root of the model 117 points = get_children_of_type("Point", model) 118 for point in points: 119 print('Point: {}'.format(point)) 120 121 # Output: 122 # Point: 5,10 123 # Point: 10,10 124 # Point: 20,20 125 # Point: 5,-7 126 # Point: 10,10 127 ``` 128 129 130 ## Video tutorials 131 132 133 ### Introduction to textX 134 135 136 [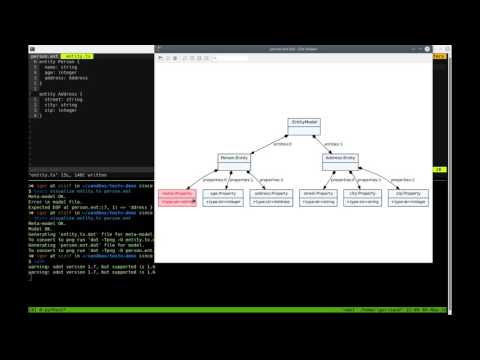](https://www.youtube.com/watch?v=CN2IVtInapo) 138 139 140 ### Implementing Martin Fowler's State Machine DSL in textX 141 142 [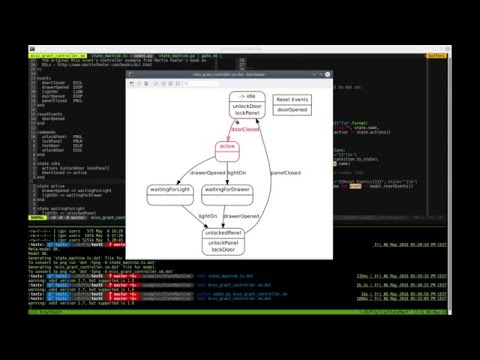](https://www.youtube.com/watch?v=HI14jk0JIR0) 144 145 146 ## Docs and tutorials 147 148 The full documentation with tutorials is available at 149 http://textx.github.io/textX/stable/ 150 151 152 # Support in IDE/editors 153 154 Projects that are currently in progress are: 155 156 - [textX-LS](https://github.com/textX/textX-LS) - support for Language Server 157 Protocol and VS Code for any textX based language. This project is about to 158 supersede the following projects: 159 - [textX-languageserver](https://github.com/textX/textX-languageserver) - 160 Language Server Protocol support for textX languages 161 - [textX-extensions](https://github.com/textX/textX-extensions) - syntax 162 highlighting, code outline 163 - [viewX](https://github.com/danielkupco/viewX-vscode) - creating visualizers 164 for textX languages 165 166 If you are a vim editor user check 167 out [support for vim](https://github.com/textX/textx.vim/). 168 169 For emacs there is [textx-mode](https://github.com/textX/textx-mode) which is 170 also available in [MELPA](https://melpa.org/#/textx-mode). 171 172 You can also check 173 out [textX-ninja project](https://github.com/textX/textX-ninja). It is 174 currently unmaintained. 175 176 177 ## Discussion and help 178 179 For general questions and help please use 180 [StackOverflow](https://stackoverflow.com/questions/tagged/textx). Just make 181 sure to tag your question with the `textx` tag. 182 183 184 For issues, suggestions and feature request please use 185 [GitHub issue tracker](https://github.com/textX/textX/issues). 186 187 188 ## Citing textX 189 190 If you are using textX in your research project we would be very grateful if you 191 cite our paper: 192 193 Dejanović I., Vaderna R., Milosavljević G., Vuković Ž. (2017). [TextX: A Python 194 tool for Domain-Specific Languages 195 implementation](https://www.doi.org/10.1016/j.knosys.2016.10.023). 196 Knowledge-Based Systems, 115, 1-4. 197 198 199 ## License 200 201 MIT 202 203 ## Python versions 204 205 Tested for 2.7, 3.4+ 206 207 208 [Arpeggio]: https://github.com/textX/Arpeggio 209 [Xtext]: http://www.eclipse.org/Xtext/ 210 211Keywords: parser,meta-language,meta-model,language,DSL 212Platform: UNKNOWN 213Classifier: Development Status :: 5 - Production/Stable 214Classifier: Intended Audience :: Developers 215Classifier: Intended Audience :: Information Technology 216Classifier: Intended Audience :: Science/Research 217Classifier: Topic :: Software Development :: Interpreters 218Classifier: Topic :: Software Development :: Compilers 219Classifier: Topic :: Software Development :: Libraries :: Python Modules 220Classifier: License :: OSI Approved :: MIT License 221Classifier: Operating System :: OS Independent 222Classifier: Programming Language :: Python :: 2 223Classifier: Programming Language :: Python :: 2.7 224Classifier: Programming Language :: Python :: 3 225Classifier: Programming Language :: Python :: 3.4 226Classifier: Programming Language :: Python :: 3.5 227Classifier: Programming Language :: Python :: 3.6 228Classifier: Programming Language :: Python :: 3.7 229Classifier: Programming Language :: Python :: 3.8 230Description-Content-Type: text/markdown 231Provides-Extra: cli 232Provides-Extra: dev 233Provides-Extra: test 234