README.md
1
2
3[](https://pypi.python.org/pypi/textX)
4
5[](https://travis-ci.org/textX/textX)
6[](https://coveralls.io/github/textX/textX?branch=master)
7[](http://textx.github.io/textX/latest/)
8
9
10textX is a meta-language for building Domain-Specific Languages (DSLs) in
11Python. It is inspired by [Xtext].
12
13In a nutshell, textX will help you build your textual language in an easy way.
14You can invent your own language or build a support for already existing textual
15language or file format.
16
17From a single language description (grammar), textX will build a parser and a
18meta-model (a.k.a. abstract syntax) for the language. See the docs for the
19details.
20
21textX follows the syntax and semantics of Xtext but [differs in some
22places](http://textx.github.io/textX/latest/about/comparison/) and is
23implemented 100% in Python using [Arpeggio] PEG parser - no grammar ambiguities,
24unlimited lookahead, interpreter style of work.
25
26
27## Quick intro
28
29Here is a complete example that shows the definition of a simple DSL for
30drawing. We also show how to define a custom class, interpret models and search
31for instances of a particular type.
32
33```python
34from textx import metamodel_from_str, get_children_of_type
35
36grammar = """
37Model: commands*=DrawCommand;
38DrawCommand: MoveCommand | ShapeCommand;
39ShapeCommand: LineTo | Circle;
40MoveCommand: MoveTo | MoveBy;
41MoveTo: 'move' 'to' position=Point;
42MoveBy: 'move' 'by' vector=Point;
43Circle: 'circle' radius=INT;
44LineTo: 'line' 'to' point=Point;
45Point: x=INT ',' y=INT;
46"""
47
48# We will provide our class for Point.
49# Classes for other rules will be dynamically generated.
50class Point(object):
51 def __init__(self, parent, x, y):
52 self.parent = parent
53 self.x = x
54 self.y = y
55
56 def __str__(self):
57 return "{},{}".format(self.x, self.y)
58
59 def __add__(self, other):
60 return Point(self.parent, self.x + other.x, self.y + other.y)
61
62# Create meta-model from the grammar. Provide `Point` class to be used for
63# the rule `Point` from the grammar.
64mm = metamodel_from_str(grammar, classes=[Point])
65
66model_str = """
67 move to 5, 10
68 line to 10, 10
69 line to 20, 20
70 move by 5, -7
71 circle 10
72 line to 10, 10
73"""
74
75# Meta-model knows how to parse and instantiate models.
76model = mm.model_from_str(model_str)
77
78# At this point model is a plain Python object graph with instances of
79# dynamically created classes and attributes following the grammar.
80
81def cname(o):
82 return o.__class__.__name__
83
84# Let's interpret the model
85position = Point(None, 0, 0)
86for command in model.commands:
87 if cname(command) == 'MoveTo':
88 print('Moving to position', command.position)
89 position = command.position
90 elif cname(command) == 'MoveBy':
91 position = position + command.vector
92 print('Moving by', command.vector, 'to a new position', position)
93 elif cname(command) == 'Circle':
94 print('Drawing circle at', position, 'with radius', command.radius)
95 else:
96 print('Drawing line from', position, 'to', command.point)
97 position = command.point
98print('End position is', position)
99
100# Output:
101# Moving to position 5,10
102# Drawing line from 5,10 to 10,10
103# Drawing line from 10,10 to 20,20
104# Moving by 5,-7 to a new position 25,13
105# Drawing circle at 25,13 with radius 10
106# Drawing line from 25,13 to 10,10
107
108# Collect all points starting from the root of the model
109points = get_children_of_type("Point", model)
110for point in points:
111 print('Point: {}'.format(point))
112
113# Output:
114# Point: 5,10
115# Point: 10,10
116# Point: 20,20
117# Point: 5,-7
118# Point: 10,10
119```
120
121
122## Video tutorials
123
124
125### Introduction to textX
126
127
128[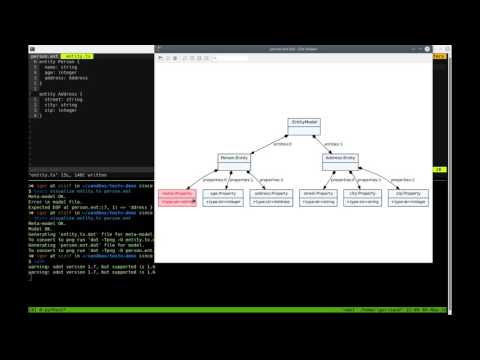](https://www.youtube.com/watch?v=CN2IVtInapo)
130
131
132### Implementing Martin Fowler's State Machine DSL in textX
133
134[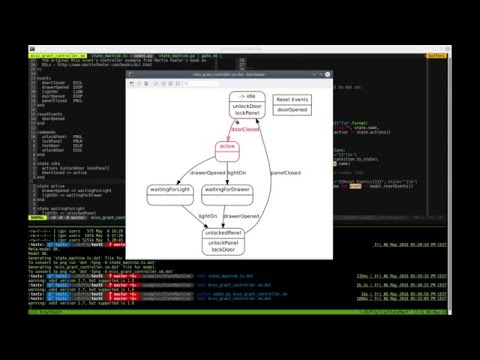](https://www.youtube.com/watch?v=HI14jk0JIR0)
136
137
138## Docs and tutorials
139
140The full documentation with tutorials is available at
141http://textx.github.io/textX/stable/
142
143
144# Support in IDE/editors
145
146Projects that are currently in progress are:
147
148- [textX-LS](https://github.com/textX/textX-LS) - support for Language Server
149 Protocol and VS Code for any textX based language. This project is about to
150 supersede the following projects:
151 - [textX-languageserver](https://github.com/textX/textX-languageserver) -
152 Language Server Protocol support for textX languages
153 - [textX-extensions](https://github.com/textX/textX-extensions) - syntax
154 highlighting, code outline
155- [viewX](https://github.com/danielkupco/viewX-vscode) - creating visualizers
156 for textX languages
157
158If you are a vim editor user check
159out [support for vim](https://github.com/textX/textx.vim/).
160
161For emacs there is [textx-mode](https://github.com/textX/textx-mode) which is
162also available in [MELPA](https://melpa.org/#/textx-mode).
163
164You can also check
165out [textX-ninja project](https://github.com/textX/textX-ninja). It is
166currently unmaintained.
167
168
169## Discussion and help
170
171For general questions and help please use
172[StackOverflow](https://stackoverflow.com/questions/tagged/textx). Just make
173sure to tag your question with the `textx` tag.
174
175
176For issues, suggestions and feature request please use
177[GitHub issue tracker](https://github.com/textX/textX/issues).
178
179
180## Citing textX
181
182If you are using textX in your research project we would be very grateful if you
183cite our paper:
184
185Dejanović I., Vaderna R., Milosavljević G., Vuković Ž. (2017). [TextX: A Python
186tool for Domain-Specific Languages
187implementation](https://www.doi.org/10.1016/j.knosys.2016.10.023).
188Knowledge-Based Systems, 115, 1-4.
189
190
191## License
192
193MIT
194
195## Python versions
196
197Tested for 2.7, 3.4+
198
199
200[Arpeggio]: https://github.com/textX/Arpeggio
201[Xtext]: http://www.eclipse.org/Xtext/
202