README.md
1draw2d
2======
3[](http://gocover.io/github.com/llgcode/draw2d)
4[](https://godoc.org/github.com/llgcode/draw2d)
5
6Package draw2d is a pure [go](http://golang.org) 2D vector graphics library with support for multiple output devices such as [images](http://golang.org/pkg/image) (draw2d), pdf documents (draw2dpdf) and opengl (draw2dgl), which can also be used on the google app engine. It can be used as a pure go [Cairo](http://www.cairographics.org/) alternative. draw2d is released under the BSD license. See the [documentation](http://godoc.org/github.com/llgcode/draw2d) for more details.
7
8[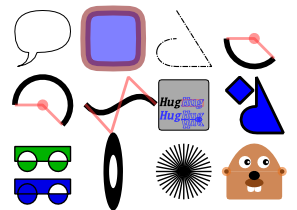](https://raw.githubusercontent.com/llgcode/draw2d/master/resource/image/geometry.pdf)[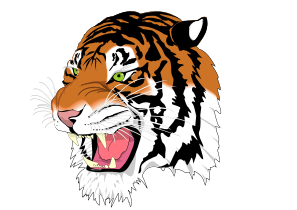](https://raw.githubusercontent.com/llgcode/draw2d/master/resource/image/postscript.pdf)
9
10Click on an image above to get the pdf, generated with exactly the same draw2d code. The first image is the output of `samples/geometry`. The second image is the result of `samples/postcript`, which demonstrates that draw2d can draw postscript files into images or pdf documents with the [ps](https://github.com/llgcode/ps) package.
11
12Features
13--------
14
15Operations in draw2d include stroking and filling polygons, arcs, Bézier curves, drawing images and text rendering with truetype fonts. All drawing operations can be transformed by affine transformations (scale, rotation, translation).
16
17Package draw2d follows the conventions of the [HTML Canvas 2D Context](http://www.w3.org/TR/2dcontext/) for coordinate system, angles, etc...
18
19Installation
20------------
21
22Install [golang](http://golang.org/doc/install). To install or update the package draw2d on your system, run:
23
24Stable release
25```
26go get -u gopkg.in/llgcode/draw2d.v1
27```
28
29or Current release
30```
31go get -u github.com/llgcode/draw2d
32```
33
34
35Quick Start
36-----------
37
38The following Go code generates a simple drawing and saves it to an image file with package draw2d:
39
40```go
41package main
42
43import (
44 "github.com/llgcode/draw2d/draw2dimg"
45 "image"
46 "image/color"
47)
48
49func main() {
50 // Initialize the graphic context on an RGBA image
51 dest := image.NewRGBA(image.Rect(0, 0, 297, 210.0))
52 gc := draw2dimg.NewGraphicContext(dest)
53
54 // Set some properties
55 gc.SetFillColor(color.RGBA{0x44, 0xff, 0x44, 0xff})
56 gc.SetStrokeColor(color.RGBA{0x44, 0x44, 0x44, 0xff})
57 gc.SetLineWidth(5)
58
59 // Draw a closed shape
60 gc.MoveTo(10, 10) // should always be called first for a new path
61 gc.LineTo(100, 50)
62 gc.QuadCurveTo(100, 10, 10, 10)
63 gc.Close()
64 gc.FillStroke()
65
66 // Save to file
67 draw2dimg.SaveToPngFile("hello.png", dest)
68}
69```
70
71The same Go code can also generate a pdf document with package draw2dpdf:
72
73```go
74package main
75
76import (
77 "github.com/llgcode/draw2d/draw2dpdf"
78 "image/color"
79)
80
81func main() {
82 // Initialize the graphic context on an RGBA image
83 dest := draw2dpdf.NewPdf("L", "mm", "A4")
84 gc := draw2dpdf.NewGraphicContext(dest)
85
86 // Set some properties
87 gc.SetFillColor(color.RGBA{0x44, 0xff, 0x44, 0xff})
88 gc.SetStrokeColor(color.RGBA{0x44, 0x44, 0x44, 0xff})
89 gc.SetLineWidth(5)
90
91 // Draw a closed shape
92 gc.MoveTo(10, 10) // should always be called first for a new path
93 gc.LineTo(100, 50)
94 gc.QuadCurveTo(100, 10, 10, 10)
95 gc.Close()
96 gc.FillStroke()
97
98 // Save to file
99 draw2dpdf.SaveToPdfFile("hello.pdf", dest)
100}
101```
102
103There are more examples here: https://github.com/llgcode/draw2d/tree/master/samples
104
105Drawing on opengl is provided by the draw2dgl package.
106
107Testing
108-------
109
110The samples are run as tests from the root package folder `draw2d` by:
111```
112go test ./...
113```
114Or if you want to run with test coverage:
115```
116go test -cover ./... | grep -v "no test"
117```
118This will generate output by the different backends in the output folder.
119
120Acknowledgments
121---------------
122
123[Laurent Le Goff](https://github.com/llgcode) wrote this library, inspired by [Postscript](http://www.tailrecursive.org/postscript) and [HTML5 canvas](http://www.w3.org/TR/2dcontext/). He implemented the image and opengl backend with the [freetype-go](https://code.google.com/p/freetype-go/) package. Also he created a pure go [Postscript interpreter](https://github.com/llgcode/ps), which can read postscript images and draw to a draw2d graphic context. [Stani Michiels](https://github.com/stanim) implemented the pdf backend with the [gofpdf](https://github.com/jung-kurt/gofpdf) package.
124
125
126
127Packages using draw2d
128---------------------
129
130 - [ps](https://github.com/llgcode/ps): Postscript interpreter written in Go
131 - [gonum/plot](https://github.com/gonum/plot): drawing plots in Go
132 - [go.uik](https://github.com/skelterjohn/go.uik): a concurrent UI kit written in pure go.
133 - [smartcrop](https://github.com/muesli/smartcrop): content aware image cropping
134 - [karta](https://github.com/peterhellberg/karta): drawing Voronoi diagrams
135 - [chart](https://github.com/vdobler/chart): basic charts in Go
136 - [hilbert](https://github.com/google/hilbert): package for drawing Hilbert curves
137
138References
139---------
140
141 - [antigrain.com](http://www.antigrain.com)
142 - [freetype-go](http://code.google.com/p/freetype-go)
143 -
144