README.md
1# promptui
2
3Interactive prompt for command-line applications.
4
5We built Promptui because we wanted to make it easy and fun to explore cloud
6services with [manifold cli](https://github.com/manifoldco/manifold-cli).
7
8[Code of Conduct](./CODE_OF_CONDUCT.md) |
9[Contribution Guidelines](./.github/CONTRIBUTING.md)
10
11[](https://github.com/manifoldco/promptui/releases)
12[](https://godoc.org/github.com/manifoldco/promptui)
13[](https://travis-ci.org/manifoldco/promptui)
14[](https://goreportcard.com/report/github.com/manifoldco/promptui)
15[](./LICENSE.md)
16
17## Overview
18
19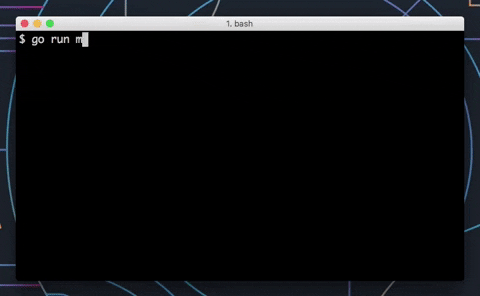
20
21Promptui is a library providing a simple interface to create command-line
22prompts for go. It can be easily integrated into
23[spf13/cobra](https://github.com/spf13/cobra),
24[urfave/cli](https://github.com/urfave/cli) or any cli go application.
25
26Promptui has two main input modes:
27
28- `Prompt` provides a single line for user input. Prompt supports
29 optional live validation, confirmation and masking the input.
30
31- `Select` provides a list of options to choose from. Select supports
32 pagination, search, detailed view and custom templates.
33
34For a full list of options check [GoDoc](https://godoc.org/github.com/manifoldco/promptui).
35
36## Basic Usage
37
38### Prompt
39
40```go
41package main
42
43import (
44 "errors"
45 "fmt"
46 "strconv"
47
48 "github.com/manifoldco/promptui"
49)
50
51func main() {
52 validate := func(input string) error {
53 _, err := strconv.ParseFloat(input, 64)
54 if err != nil {
55 return errors.New("Invalid number")
56 }
57 return nil
58 }
59
60 prompt := promptui.Prompt{
61 Label: "Number",
62 Validate: validate,
63 }
64
65 result, err := prompt.Run()
66
67 if err != nil {
68 fmt.Printf("Prompt failed %v\n", err)
69 return
70 }
71
72 fmt.Printf("You choose %q\n", result)
73}
74```
75
76### Select
77
78```go
79package main
80
81import (
82 "fmt"
83
84 "github.com/manifoldco/promptui"
85)
86
87func main() {
88 prompt := promptui.Select{
89 Label: "Select Day",
90 Items: []string{"Monday", "Tuesday", "Wednesday", "Thursday", "Friday",
91 "Saturday", "Sunday"},
92 }
93
94 _, result, err := prompt.Run()
95
96 if err != nil {
97 fmt.Printf("Prompt failed %v\n", err)
98 return
99 }
100
101 fmt.Printf("You choose %q\n", result)
102}
103```
104
105### More Examples
106
107See full list of [examples](https://github.com/manifoldco/promptui/tree/master/_examples)
108