README.md
1Go Trello API
2================
3
4[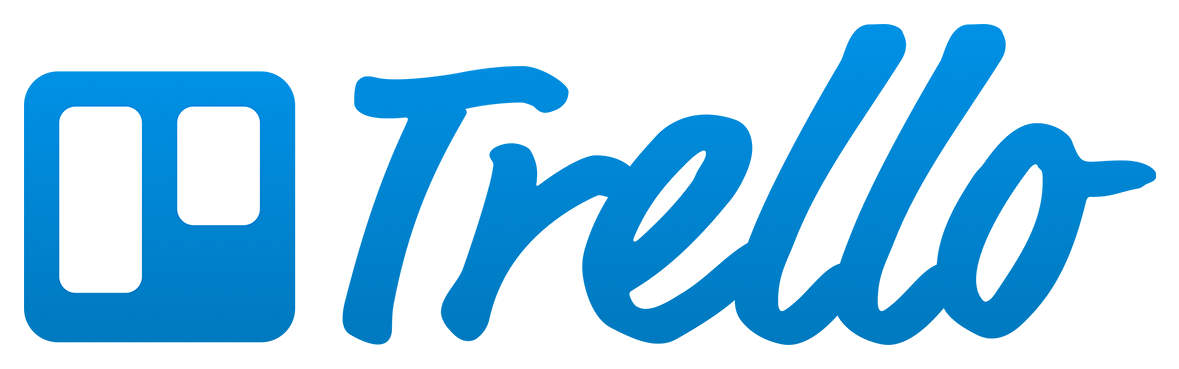](https://www.trello.com)
5
6[](http://godoc.org/github.com/adlio/trello)
7[](https://travis-ci.org/adlio/trello)
8[](https://coveralls.io/github/adlio/trello?branch=master)
9
10A #golang package to access the [Trello API](https://developers.trello.com/v1.0/reference). Nearly 100% of the
11read-only surface area of the API is covered, as is creation and modification of Cards.
12Low-level infrastructure for features to modify Lists and Boards are easy to add... just not
13done yet.
14
15Pull requests are welcome for missing features.
16
17My current development focus is documentation, especially enhancing this README.md with more
18example use cases.
19
20## Installation
21
22The Go Trello API has been Tested compatible with Go 1.7 on up. Its only dependency is
23the `github.com/pkg/errors` package. It otherwise relies only on the Go standard library.
24
25```
26go get github.com/adlio/trello
27```
28
29## Basic Usage
30
31All interaction starts with a `trello.Client`. Create one with your appKey and token:
32
33```Go
34client := trello.NewClient(appKey, token)
35```
36
37All API requests accept a trello.Arguments object. This object is a simple
38`map[string]string`, converted to query string arguments in the API call.
39Trello has sane defaults on API calls. We have a `trello.Defaults()` utility function
40which can be used when you desire the default Trello arguments. Internally,
41`trello.Defaults()` is an empty map, which translates to an empty query string.
42
43```Go
44board, err := client.GetBoard("bOaRdID", trello.Defaults())
45if err != nil {
46 // Handle error
47}
48```
49
50## Client Longevity
51
52When getting Lists from Boards or Cards from Lists, the original `trello.Client` pointer
53is carried along in returned child objects. It's important to realize that this enables
54you to make additional API calls via functions invoked on the objects you receive:
55
56```Go
57client := trello.NewClient(appKey, token)
58board, err := client.GetBoard("ID", trello.Defaults())
59
60// GetLists makes an API call to /boards/:id/lists using credentials from `client`
61lists, err := board.GetLists(trello.Defaults())
62
63for _, list := range lists {
64 // GetCards makes an API call to /lists/:id/cards using credentials from `client`
65 cards, err := list.GetCards(trello.Defaults())
66}
67```
68
69## Get Trello Boards for a User
70
71Boards can be retrieved directly by their ID (see example above), or by asking
72for all boards for a member:
73
74```Go
75member, err := client.GetMember("usernameOrId", trello.Defaults())
76if err != nil {
77 // Handle error
78}
79
80boards, err := member.GetBoards(trello.Defaults())
81if err != nil {
82 // Handle error
83}
84```
85
86## Get Trello Lists on a Board
87
88```Go
89board, err := client.GetBoard("bOaRdID", trello.Defaults())
90if err != nil {
91 // Handle error
92}
93
94lists, err := board.GetLists(trello.Defaults())
95if err != nil {
96 // Handle error
97}
98```
99
100## Get Trello Cards on a Board
101
102```Go
103board, err := client.GetBoard("bOaRdID", trello.Defaults())
104if err != nil {
105 // Handle error
106}
107
108cards, err := board.GetCards(trello.Defaults())
109if err != nil {
110 // Handle error
111}
112```
113
114## Get Trello Cards on a List
115
116```Go
117list, err := client.GetList("lIsTID", trello.Defaults())
118if err != nil {
119 // Handle error
120}
121
122cards, err := list.GetCards(trello.Defaults())
123if err != nil {
124 // Handle error
125}
126```
127
128## Creating and deleting a Board
129
130A board can be created or deleted on the `Board` struct for the user whose credentials are being used.
131
132```Go
133 board := trello.NewBoard("My bucket list")
134
135 // POST
136 err := client.CreateBoard(&board, trello.Defaults())
137
138 // DELETE
139 err := board.Delete(trello.Defaults())
140 if err != nil {
141 fmt.Println(err)
142 }
143}
144```
145
146## Creating a Card
147
148The API provides several mechanisms for creating new cards.
149
150### Creating Cards from Scratch on the Client
151
152This approach requires the most input data on the card:
153
154```Go
155card := trello.Card{
156 Name: "Card Name",
157 Desc: "Card description",
158 Pos: 12345.678,
159 IDList: "iDOfaLiSt",
160 IDLabels: []string{"labelID1", "labelID2"},
161}
162err := client.CreateCard(card, trello.Defaults())
163```
164
165
166### Creating Cards On a List
167
168```Go
169list, err := client.GetList("lIsTID", trello.Defaults())
170list.AddCard(&trello.Card{ Name: "Card Name", Desc: "Card description" }, trello.Defaults())
171```
172
173### Creating a Card by Copying Another Card
174
175```Go
176err := card.CopyToList("listIdNUmber", trello.Defaults())
177```
178
179## Get Actions on a Board
180
181```Go
182board, err := client.GetBoard("bOaRdID", trello.Defaults())
183if err != nil {
184 // Handle error
185}
186
187actions, err := board.GetActions(trello.Defaults())
188if err != nil {
189 // Handle error
190}
191```
192
193## Get Actions on a Card
194
195```Go
196card, err := client.GetCard("cArDID", trello.Defaults())
197if err != nil {
198 // Handle error
199}
200
201actions, err := card.GetActions(trello.Defaults())
202if err != nil {
203 // Handle error
204}
205```
206
207## Rearrange Cards Within a List
208
209```Go
210err := card.MoveToTopOfList()
211err = card.MoveToBottomOfList()
212err = card.SetPos(12345.6789)
213```
214
215
216## Moving a Card to Another List
217
218```Go
219err := card.MoveToList("listIdNUmber", trello.Defaults())
220```
221
222
223## Card Ancestry
224
225Trello provides ancestry tracking when cards are created as copies of other cards. This package
226provides functions for working with this data:
227
228```Go
229
230// ancestors will hold a slice of *trello.Cards, with the first
231// being the card's parent, and the last being parent's parent's parent...
232ancestors, err := card.GetAncestorCards(trello.Defaults())
233
234// GetOriginatingCard() is an alias for the last element in the slice
235// of ancestor cards.
236ultimateParentCard, err := card.GetOriginatingCard(trello.Defaults())
237
238```
239
240## Debug Logging
241
242If you'd like to see all API calls logged, you can attach a `.Logger` (implementing `Debugf(string, ...interface{})`)
243to your client. The interface for the logger mimics logrus. Example usage:
244
245```Go
246logger := logrus.New()
247logger.SetLevel(logrus.DebugLevel)
248client := trello.NewClient(appKey, token)
249client.Logger = logger
250```
251