1# buffer [![travis][travis-image]][travis-url] [![npm][npm-image]][npm-url] [![downloads][downloads-image]][npm-url] 2 3#### The buffer module from [node.js](http://nodejs.org/), for the browser. 4 5[![saucelabs][saucelabs-image]][saucelabs-url] 6 7[travis-image]: https://img.shields.io/travis/feross/buffer.svg?style=flat 8[travis-url]: https://travis-ci.org/feross/buffer 9[npm-image]: https://img.shields.io/npm/v/buffer.svg?style=flat 10[npm-url]: https://npmjs.org/package/buffer 11[downloads-image]: https://img.shields.io/npm/dm/buffer.svg?style=flat 12[saucelabs-image]: https://saucelabs.com/browser-matrix/buffer.svg 13[saucelabs-url]: https://saucelabs.com/u/buffer 14 15With [browserify](http://browserify.org), simply `require('buffer')` or use the `Buffer` global and you will get this module. 16 17The goal is to provide an API that is 100% identical to 18[node's Buffer API](http://iojs.org/api/buffer.html). Read the 19[official docs](http://iojs.org/api/buffer.html) for the full list of properties, 20instance methods, and class methods that are supported. 21 22## features 23 24- Manipulate binary data like a boss, in all browsers -- even IE6! 25- Super fast. Backed by Typed Arrays (`Uint8Array`/`ArrayBuffer`, not `Object`) 26- Extremely small bundle size (**5.04KB minified + gzipped**, 35.5KB with comments) 27- Excellent browser support (IE 6+, Chrome 4+, Firefox 3+, Safari 5.1+, Opera 11+, iOS, etc.) 28- Preserves Node API exactly, with one important difference (see below) 29- `.slice()` returns instances of the same type (Buffer) 30- Square-bracket `buf[4]` notation works, even in old browsers like IE6! 31- Does not modify any browser prototypes or put anything on `window` 32- Comprehensive test suite (including all buffer tests from node.js core) 33 34 35## install 36 37To use this module directly (without browserify), install it: 38 39```bash 40npm install buffer 41``` 42 43This module was previously called **native-buffer-browserify**, but please use **buffer** 44from now on. 45 46A standalone bundle is available [here](https://wzrd.in/standalone/buffer), for non-browserify users. 47 48 49## usage 50 51The module's API is identical to node's `Buffer` API. Read the 52[official docs](http://iojs.org/api/buffer.html) for the full list of properties, 53instance methods, and class methods that are supported. 54 55As mentioned above, `require('buffer')` or use the `Buffer` global with 56[browserify](http://browserify.org) and this module will automatically be included 57in your bundle. Almost any npm module will work in the browser, even if it assumes that 58the node `Buffer` API will be available. 59 60To depend on this module explicitly (without browserify), require it like this: 61 62```js 63var Buffer = require('buffer/').Buffer // note: the trailing slash is important! 64``` 65 66To require this module explicitly, use `require('buffer/')` which tells the node.js module 67lookup algorithm (also used by browserify) to use the **npm module** named `buffer` 68instead of the **node.js core** module named `buffer`! 69 70 71## how does it work? 72 73`Buffer` is a subclass of `Uint8Array` augmented with all the `Buffer` API methods. 74The `Uint8Array` prototype is not modified. 75 76 77## one minor difference 78 79#### In old browsers, `buf.slice()` does not modify parent buffer's memory 80 81If you only support modern browsers (specifically, those with typed array support), 82then this issue does not affect you. If you support super old browsers, then read on. 83 84In node, the `slice()` method returns a new `Buffer` that shares underlying memory 85with the original Buffer. When you modify one buffer, you modify the other. 86[Read more.](http://iojs.org/api/buffer.html#buffer_buf_slice_start_end) 87 88In browsers with typed array support, this `Buffer` implementation supports this 89behavior. In browsers without typed arrays, an alternate buffer implementation is 90used that is based on `Object` which has no mechanism to point separate 91`Buffer`s to the same underlying slab of memory. 92 93You can see which browser versions lack typed array support 94[here](https://github.com/feross/buffer/blob/master/index.js#L20-L46). 95 96 97## tracking the latest node api 98 99This module tracks the Buffer API in the latest (unstable) version of node.js. The Buffer 100API is considered **stable** in the 101[node stability index](http://nodejs.org/docs/latest/api/documentation.html#documentation_stability_index), 102so it is unlikely that there will ever be breaking changes. 103Nonetheless, when/if the Buffer API changes in node, this module's API will change 104accordingly. 105 106## performance 107 108See perf tests in `/perf`. 109 110`BrowserBuffer` is the browser `buffer` module (this repo). `Uint8Array` is included as a 111sanity check (since `BrowserBuffer` uses `Uint8Array` under the hood, `Uint8Array` will 112always be at least a bit faster). Finally, `NodeBuffer` is the node.js buffer module, 113which is included to compare against. 114 115### Chrome 38 116 117| Method | Operations | Accuracy | Sampled | Fastest | 118|:-------|:-----------|:---------|:--------|:-------:| 119| BrowserBuffer#bracket-notation | 11,457,464 ops/sec | ±0.86% | 66 | ✓ | 120| Uint8Array#bracket-notation | 10,824,332 ops/sec | ±0.74% | 65 | | 121| | | | | 122| BrowserBuffer#concat | 450,532 ops/sec | ±0.76% | 68 | | 123| Uint8Array#concat | 1,368,911 ops/sec | ±1.50% | 62 | ✓ | 124| | | | | 125| BrowserBuffer#copy(16000) | 903,001 ops/sec | ±0.96% | 67 | | 126| Uint8Array#copy(16000) | 1,422,441 ops/sec | ±1.04% | 66 | ✓ | 127| | | | | 128| BrowserBuffer#copy(16) | 11,431,358 ops/sec | ±0.46% | 69 | | 129| Uint8Array#copy(16) | 13,944,163 ops/sec | ±1.12% | 68 | ✓ | 130| | | | | 131| BrowserBuffer#new(16000) | 106,329 ops/sec | ±6.70% | 44 | | 132| Uint8Array#new(16000) | 131,001 ops/sec | ±2.85% | 31 | ✓ | 133| | | | | 134| BrowserBuffer#new(16) | 1,554,491 ops/sec | ±1.60% | 65 | | 135| Uint8Array#new(16) | 6,623,930 ops/sec | ±1.66% | 65 | ✓ | 136| | | | | 137| BrowserBuffer#readDoubleBE | 112,830 ops/sec | ±0.51% | 69 | ✓ | 138| DataView#getFloat64 | 93,500 ops/sec | ±0.57% | 68 | | 139| | | | | 140| BrowserBuffer#readFloatBE | 146,678 ops/sec | ±0.95% | 68 | ✓ | 141| DataView#getFloat32 | 99,311 ops/sec | ±0.41% | 67 | | 142| | | | | 143| BrowserBuffer#readUInt32LE | 843,214 ops/sec | ±0.70% | 69 | ✓ | 144| DataView#getUint32 | 103,024 ops/sec | ±0.64% | 67 | | 145| | | | | 146| BrowserBuffer#slice | 1,013,941 ops/sec | ±0.75% | 67 | | 147| Uint8Array#subarray | 1,903,928 ops/sec | ±0.53% | 67 | ✓ | 148| | | | | 149| BrowserBuffer#writeFloatBE | 61,387 ops/sec | ±0.90% | 67 | | 150| DataView#setFloat32 | 141,249 ops/sec | ±0.40% | 66 | ✓ | 151 152 153### Firefox 33 154 155| Method | Operations | Accuracy | Sampled | Fastest | 156|:-------|:-----------|:---------|:--------|:-------:| 157| BrowserBuffer#bracket-notation | 20,800,421 ops/sec | ±1.84% | 60 | | 158| Uint8Array#bracket-notation | 20,826,235 ops/sec | ±2.02% | 61 | ✓ | 159| | | | | 160| BrowserBuffer#concat | 153,076 ops/sec | ±2.32% | 61 | | 161| Uint8Array#concat | 1,255,674 ops/sec | ±8.65% | 52 | ✓ | 162| | | | | 163| BrowserBuffer#copy(16000) | 1,105,312 ops/sec | ±1.16% | 63 | | 164| Uint8Array#copy(16000) | 1,615,911 ops/sec | ±0.55% | 66 | ✓ | 165| | | | | 166| BrowserBuffer#copy(16) | 16,357,599 ops/sec | ±0.73% | 68 | | 167| Uint8Array#copy(16) | 31,436,281 ops/sec | ±1.05% | 68 | ✓ | 168| | | | | 169| BrowserBuffer#new(16000) | 52,995 ops/sec | ±6.01% | 35 | | 170| Uint8Array#new(16000) | 87,686 ops/sec | ±5.68% | 45 | ✓ | 171| | | | | 172| BrowserBuffer#new(16) | 252,031 ops/sec | ±1.61% | 66 | | 173| Uint8Array#new(16) | 8,477,026 ops/sec | ±0.49% | 68 | ✓ | 174| | | | | 175| BrowserBuffer#readDoubleBE | 99,871 ops/sec | ±0.41% | 69 | | 176| DataView#getFloat64 | 285,663 ops/sec | ±0.70% | 68 | ✓ | 177| | | | | 178| BrowserBuffer#readFloatBE | 115,540 ops/sec | ±0.42% | 69 | | 179| DataView#getFloat32 | 288,722 ops/sec | ±0.82% | 68 | ✓ | 180| | | | | 181| BrowserBuffer#readUInt32LE | 633,926 ops/sec | ±1.08% | 67 | ✓ | 182| DataView#getUint32 | 294,808 ops/sec | ±0.79% | 64 | | 183| | | | | 184| BrowserBuffer#slice | 349,425 ops/sec | ±0.46% | 69 | | 185| Uint8Array#subarray | 5,965,819 ops/sec | ±0.60% | 65 | ✓ | 186| | | | | 187| BrowserBuffer#writeFloatBE | 59,980 ops/sec | ±0.41% | 67 | | 188| DataView#setFloat32 | 317,634 ops/sec | ±0.63% | 68 | ✓ | 189 190### Safari 8 191 192| Method | Operations | Accuracy | Sampled | Fastest | 193|:-------|:-----------|:---------|:--------|:-------:| 194| BrowserBuffer#bracket-notation | 10,279,729 ops/sec | ±2.25% | 56 | ✓ | 195| Uint8Array#bracket-notation | 10,030,767 ops/sec | ±2.23% | 59 | | 196| | | | | 197| BrowserBuffer#concat | 144,138 ops/sec | ±1.38% | 65 | | 198| Uint8Array#concat | 4,950,764 ops/sec | ±1.70% | 63 | ✓ | 199| | | | | 200| BrowserBuffer#copy(16000) | 1,058,548 ops/sec | ±1.51% | 64 | | 201| Uint8Array#copy(16000) | 1,409,666 ops/sec | ±1.17% | 65 | ✓ | 202| | | | | 203| BrowserBuffer#copy(16) | 6,282,529 ops/sec | ±1.88% | 58 | | 204| Uint8Array#copy(16) | 11,907,128 ops/sec | ±2.87% | 58 | ✓ | 205| | | | | 206| BrowserBuffer#new(16000) | 101,663 ops/sec | ±3.89% | 57 | | 207| Uint8Array#new(16000) | 22,050,818 ops/sec | ±6.51% | 46 | ✓ | 208| | | | | 209| BrowserBuffer#new(16) | 176,072 ops/sec | ±2.13% | 64 | | 210| Uint8Array#new(16) | 24,385,731 ops/sec | ±5.01% | 51 | ✓ | 211| | | | | 212| BrowserBuffer#readDoubleBE | 41,341 ops/sec | ±1.06% | 67 | | 213| DataView#getFloat64 | 322,280 ops/sec | ±0.84% | 68 | ✓ | 214| | | | | 215| BrowserBuffer#readFloatBE | 46,141 ops/sec | ±1.06% | 65 | | 216| DataView#getFloat32 | 337,025 ops/sec | ±0.43% | 69 | ✓ | 217| | | | | 218| BrowserBuffer#readUInt32LE | 151,551 ops/sec | ±1.02% | 66 | | 219| DataView#getUint32 | 308,278 ops/sec | ±0.94% | 67 | ✓ | 220| | | | | 221| BrowserBuffer#slice | 197,365 ops/sec | ±0.95% | 66 | | 222| Uint8Array#subarray | 9,558,024 ops/sec | ±3.08% | 58 | ✓ | 223| | | | | 224| BrowserBuffer#writeFloatBE | 17,518 ops/sec | ±1.03% | 63 | | 225| DataView#setFloat32 | 319,751 ops/sec | ±0.48% | 68 | ✓ | 226 227 228### Node 0.11.14 229 230| Method | Operations | Accuracy | Sampled | Fastest | 231|:-------|:-----------|:---------|:--------|:-------:| 232| BrowserBuffer#bracket-notation | 10,489,828 ops/sec | ±3.25% | 90 | | 233| Uint8Array#bracket-notation | 10,534,884 ops/sec | ±0.81% | 92 | ✓ | 234| NodeBuffer#bracket-notation | 10,389,910 ops/sec | ±0.97% | 87 | | 235| | | | | 236| BrowserBuffer#concat | 487,830 ops/sec | ±2.58% | 88 | | 237| Uint8Array#concat | 1,814,327 ops/sec | ±1.28% | 88 | ✓ | 238| NodeBuffer#concat | 1,636,523 ops/sec | ±1.88% | 73 | | 239| | | | | 240| BrowserBuffer#copy(16000) | 1,073,665 ops/sec | ±0.77% | 90 | | 241| Uint8Array#copy(16000) | 1,348,517 ops/sec | ±0.84% | 89 | ✓ | 242| NodeBuffer#copy(16000) | 1,289,533 ops/sec | ±0.82% | 93 | | 243| | | | | 244| BrowserBuffer#copy(16) | 12,782,706 ops/sec | ±0.74% | 85 | | 245| Uint8Array#copy(16) | 14,180,427 ops/sec | ±0.93% | 92 | ✓ | 246| NodeBuffer#copy(16) | 11,083,134 ops/sec | ±1.06% | 89 | | 247| | | | | 248| BrowserBuffer#new(16000) | 141,678 ops/sec | ±3.30% | 67 | | 249| Uint8Array#new(16000) | 161,491 ops/sec | ±2.96% | 60 | | 250| NodeBuffer#new(16000) | 292,699 ops/sec | ±3.20% | 55 | ✓ | 251| | | | | 252| BrowserBuffer#new(16) | 1,655,466 ops/sec | ±2.41% | 82 | | 253| Uint8Array#new(16) | 14,399,926 ops/sec | ±0.91% | 94 | ✓ | 254| NodeBuffer#new(16) | 3,894,696 ops/sec | ±0.88% | 92 | | 255| | | | | 256| BrowserBuffer#readDoubleBE | 109,582 ops/sec | ±0.75% | 93 | ✓ | 257| DataView#getFloat64 | 91,235 ops/sec | ±0.81% | 90 | | 258| NodeBuffer#readDoubleBE | 88,593 ops/sec | ±0.96% | 81 | | 259| | | | | 260| BrowserBuffer#readFloatBE | 139,854 ops/sec | ±1.03% | 85 | ✓ | 261| DataView#getFloat32 | 98,744 ops/sec | ±0.80% | 89 | | 262| NodeBuffer#readFloatBE | 92,769 ops/sec | ±0.94% | 93 | | 263| | | | | 264| BrowserBuffer#readUInt32LE | 710,861 ops/sec | ±0.82% | 92 | | 265| DataView#getUint32 | 117,893 ops/sec | ±0.84% | 91 | | 266| NodeBuffer#readUInt32LE | 851,412 ops/sec | ±0.72% | 93 | ✓ | 267| | | | | 268| BrowserBuffer#slice | 1,673,877 ops/sec | ±0.73% | 94 | | 269| Uint8Array#subarray | 6,919,243 ops/sec | ±0.67% | 90 | ✓ | 270| NodeBuffer#slice | 4,617,604 ops/sec | ±0.79% | 93 | | 271| | | | | 272| BrowserBuffer#writeFloatBE | 66,011 ops/sec | ±0.75% | 93 | | 273| DataView#setFloat32 | 127,760 ops/sec | ±0.72% | 93 | ✓ | 274| NodeBuffer#writeFloatBE | 103,352 ops/sec | ±0.83% | 93 | | 275 276### iojs 1.8.1 277 278| Method | Operations | Accuracy | Sampled | Fastest | 279|:-------|:-----------|:---------|:--------|:-------:| 280| BrowserBuffer#bracket-notation | 10,990,488 ops/sec | ±1.11% | 91 | | 281| Uint8Array#bracket-notation | 11,268,757 ops/sec | ±0.65% | 97 | | 282| NodeBuffer#bracket-notation | 11,353,260 ops/sec | ±0.83% | 94 | ✓ | 283| | | | | 284| BrowserBuffer#concat | 378,954 ops/sec | ±0.74% | 94 | | 285| Uint8Array#concat | 1,358,288 ops/sec | ±0.97% | 87 | | 286| NodeBuffer#concat | 1,934,050 ops/sec | ±1.11% | 78 | ✓ | 287| | | | | 288| BrowserBuffer#copy(16000) | 894,538 ops/sec | ±0.56% | 84 | | 289| Uint8Array#copy(16000) | 1,442,656 ops/sec | ±0.71% | 96 | | 290| NodeBuffer#copy(16000) | 1,457,898 ops/sec | ±0.53% | 92 | ✓ | 291| | | | | 292| BrowserBuffer#copy(16) | 12,870,457 ops/sec | ±0.67% | 95 | | 293| Uint8Array#copy(16) | 16,643,989 ops/sec | ±0.61% | 93 | ✓ | 294| NodeBuffer#copy(16) | 14,885,848 ops/sec | ±0.74% | 94 | | 295| | | | | 296| BrowserBuffer#new(16000) | 109,264 ops/sec | ±4.21% | 63 | | 297| Uint8Array#new(16000) | 138,916 ops/sec | ±1.87% | 61 | | 298| NodeBuffer#new(16000) | 281,449 ops/sec | ±3.58% | 51 | ✓ | 299| | | | | 300| BrowserBuffer#new(16) | 1,362,935 ops/sec | ±0.56% | 99 | | 301| Uint8Array#new(16) | 6,193,090 ops/sec | ±0.64% | 95 | ✓ | 302| NodeBuffer#new(16) | 4,745,425 ops/sec | ±1.56% | 90 | | 303| | | | | 304| BrowserBuffer#readDoubleBE | 118,127 ops/sec | ±0.59% | 93 | ✓ | 305| DataView#getFloat64 | 107,332 ops/sec | ±0.65% | 91 | | 306| NodeBuffer#readDoubleBE | 116,274 ops/sec | ±0.94% | 95 | | 307| | | | | 308| BrowserBuffer#readFloatBE | 150,326 ops/sec | ±0.58% | 95 | ✓ | 309| DataView#getFloat32 | 110,541 ops/sec | ±0.57% | 98 | | 310| NodeBuffer#readFloatBE | 121,599 ops/sec | ±0.60% | 87 | | 311| | | | | 312| BrowserBuffer#readUInt32LE | 814,147 ops/sec | ±0.62% | 93 | | 313| DataView#getUint32 | 137,592 ops/sec | ±0.64% | 90 | | 314| NodeBuffer#readUInt32LE | 931,650 ops/sec | ±0.71% | 96 | ✓ | 315| | | | | 316| BrowserBuffer#slice | 878,590 ops/sec | ±0.68% | 93 | | 317| Uint8Array#subarray | 2,843,308 ops/sec | ±1.02% | 90 | | 318| NodeBuffer#slice | 4,998,316 ops/sec | ±0.68% | 90 | ✓ | 319| | | | | 320| BrowserBuffer#writeFloatBE | 65,927 ops/sec | ±0.74% | 93 | | 321| DataView#setFloat32 | 139,823 ops/sec | ±0.97% | 89 | ✓ | 322| NodeBuffer#writeFloatBE | 135,763 ops/sec | ±0.65% | 96 | | 323| | | | | 324 325## Testing the project 326 327First, install the project: 328 329 npm install 330 331Then, to run tests in Node.js, run: 332 333 npm run test-node 334 335To test locally in a browser, you can run: 336 337 npm run test-browser-local 338 339This will print out a URL that you can then open in a browser to run the tests, using [Zuul](https://github.com/defunctzombie/zuul). 340 341To run automated browser tests using Saucelabs, ensure that your `SAUCE_USERNAME` and `SAUCE_ACCESS_KEY` environment variables are set, then run: 342 343 npm test 344 345This is what's run in Travis, to check against various browsers. The list of browsers is kept in the `.zuul.yml` file. 346 347## JavaScript Standard Style 348 349This module uses [JavaScript Standard Style](https://github.com/feross/standard). 350 351[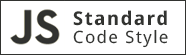](https://github.com/feross/standard) 352 353To test that the code conforms to the style, `npm install` and run: 354 355 ./node_modules/.bin/standard 356 357## credit 358 359This was originally forked from [buffer-browserify](https://github.com/toots/buffer-browserify). 360 361 362## license 363 364MIT. Copyright (C) [Feross Aboukhadijeh](http://feross.org), and other contributors. Originally forked from an MIT-licensed module by Romain Beauxis. 365