README.md
1# Word Cloud Layout
2
3This is a [Wordle](http://www.wordle.net/)-inspired word cloud layout written
4in JavaScript. It uses HTML5 canvas and sprite masks to achieve
5near-interactive speeds.
6
7See [here](http://www.jasondavies.com/wordcloud/) for an interactive
8demonstration along with implementation details.
9
10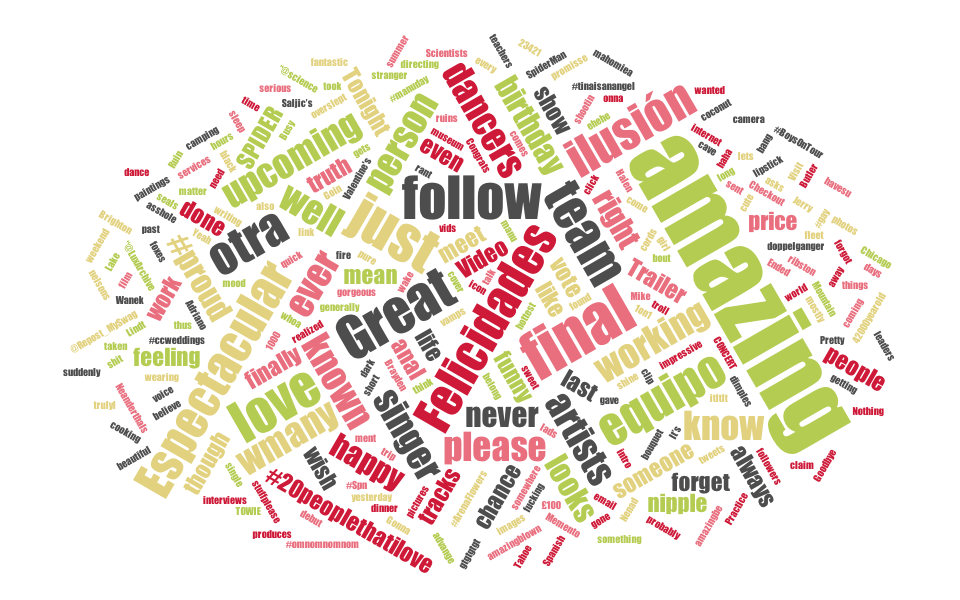
11
12## Usage
13
14See the samples in `examples/`.
15
16## API Reference
17
18<a name="cloud" href="#cloud">#</a> d3.layout.<b>cloud</b>()
19
20Constructs a new cloud layout instance.
21
22<a name="on" href="#on">#</a> <b>on</b>(<i>type</i>, <i>listener</i>)
23
24Registers the specified *listener* to receive events of the specified *type*
25from the layout. Currently, only "word" and "end" events are supported.
26
27A "word" event is dispatched every time a word is successfully placed.
28Registered listeners are called with a single argument: the word object that
29has been placed.
30
31An "end" event is dispatched when the layout has finished attempting to place
32all words. Registered listeners are called with two arguments: an array of the
33word objects that were successfully placed, and a *bounds* object of the form
34`[{x0, y0}, {x1, y1}]` representing the extent of the placed objects.
35
36<a name="start" href="#start">#</a> <b>start</b>()
37
38Starts the layout algorithm. This initialises various attributes on the word
39objects, and attempts to place each word, starting with the largest word.
40Starting with the centre of the rectangular area, each word is tested for
41collisions with all previously-placed words. If a collision is found, it tries
42to place the word in a new position along the spiral.
43
44**Note:** if a word cannot be placed in any of the positions attempted along
45the spiral, it is **not included** in the final word layout. This may be
46addressed in a future release.
47
48<a name="stop" href="#stop">#</a> <b>stop</b>()
49
50Stops the layout algorithm.
51
52<a name="timeInterval" href="#timeInterval">#</a> <b>timeInterval</b>([<i>time</i>])
53
54Internally, the layout uses `setInterval` to avoid locking up the browser’s
55event loop. If specified, **time** is the maximum amount of time that can be
56spent during the current timestep. If not specified, returns the current
57maximum time interval, which defaults to `Infinity`.
58
59<a name="words" href="#words">#</a> <b>words</b>([<i>words</i>])
60
61If specified, sets the **words** array. If not specified, returns the current
62words array, which defaults to `[]`.
63
64<a name="size" href="#size">#</a> <b>size</b>([<i>size</i>])
65
66If specified, sets the rectangular `[width, height]` of the layout. If not
67specified, returns the current size, which defaults to `[1, 1]`.
68
69<a name="font" href="#font">#</a> <b>font</b>([<i>font</i>])
70
71If specified, sets the **font** accessor function, which indicates the font
72face for each word. If not specified, returns the current font accessor
73function, which defaults to `"serif"`.
74A constant may be specified instead of a function.
75
76<a name="fontStyle" href="#fontStyle">#</a> <b>fontStyle</b>([<i>fontStyle</i>])
77
78If specified, sets the **fontStyle** accessor function, which indicates the
79font style for each word. If not specified, returns the current fontStyle
80accessor function, which defaults to `"normal"`.
81A constant may be specified instead of a function.
82
83<a name="fontWeight" href="#fontWeight">#</a> <b>fontWeight</b>([<i>fontWeight</i>])
84
85If specified, sets the **fontWeight** accessor function, which indicates the
86font weight for each word. If not specified, returns the current fontWeight
87accessor function, which defaults to `"normal"`.
88A constant may be specified instead of a function.
89
90<a name="fontSize" href="#fontSize">#</a> <b>fontSize</b>([<i>fontSize</i>])
91
92If specified, sets the **fontSize** accessor function, which indicates the
93numerical font size for each word. If not specified, returns the current
94fontSize accessor function, which defaults to:
95
96```js
97function(d) { return Math.sqrt(d.value); }
98```
99
100A constant may be specified instead of a function.
101
102<a name="rotate" href="#rotate">#</a> <b>rotate</b>([<i>rotate</i>])
103
104If specified, sets the **rotate** accessor function, which indicates the
105rotation angle (in degrees) for each word. If not specified, returns the
106current rotate accessor function, which defaults to:
107
108```js
109function() { return (~~(Math.random() * 6) - 3) * 30; }
110```
111
112A constant may be specified instead of a function.
113
114<a name="text" href="#text">#</a> <b>text</b>([<i>text</i>])
115
116If specified, sets the **text** accessor function, which indicates the text for
117each word. If not specified, returns the current text accessor function, which
118defaults to:
119
120```js
121function(d) { return d.text; }
122```
123
124A constant may be specified instead of a function.
125
126<a name="spiral" href="#spiral">#</a> <b>spiral</b>([<i>spiral</i>])
127
128If specified, sets the current type of spiral used for positioning words. This
129can either be one of the two built-in spirals, "archimedean" and "rectangular",
130or an arbitrary spiral generator can be used, of the following form:
131
132```js
133// size is the [width, height] array specified in cloud.size
134function(size) {
135 // t indicates the current step along the spiral; it may monotonically
136 // increase or decrease indicating clockwise or counterclockwise motion.
137 return function(t) { return [x, y]; };
138}
139```
140
141If not specified, returns the current spiral generator, which defaults to the
142built-in "archimedean" spiral.
143
144<a name="padding" href="#padding">#</a> <b>padding</b>([<i>padding</i>])
145
146If specified, sets the **padding** accessor function, which indicates the
147numerical padding for each word. If not specified, returns the current
148padding, which defaults to 1.
149
150<a name="random" href="#random">#</a> <b>random</b>([<i>random</i>])
151
152If specified, sets the internal random number generator, used for selecting the
153initial position of each word, and the clockwise/counterclockwise direction of
154the spiral for each word. This should return a number in the range `[0, 1)`.
155
156If not specified, returns the current random number generator, which defaults
157to `Math.random`.
158
159<a name="canvas" href="#canvas">#</a> <b>canvas</b>([<i>canvas</i>])
160
161If specified, sets the **canvas** generator function, which is used internally
162to draw text. If not specified, returns the current generator function, which
163defaults to:
164
165```js
166function() { return document.createElement("canvas"); }
167```
168
169When using Node.js, you will almost definitely override this default, e.g.
170using the [canvas module](https://www.npmjs.com/package/canvas).
171