1# colors.js 2[](https://travis-ci.org/Marak/colors.js) 3[](https://www.npmjs.org/package/colors) 4[](https://david-dm.org/Marak/colors.js) 5[](https://david-dm.org/Marak/colors.js#info=devDependencies) 6 7Please check out the [roadmap](ROADMAP.md) for upcoming features and releases. Please open Issues to provide feedback, and check the `develop` branch for the latest bleeding-edge updates. 8 9## get color and style in your node.js console 10 11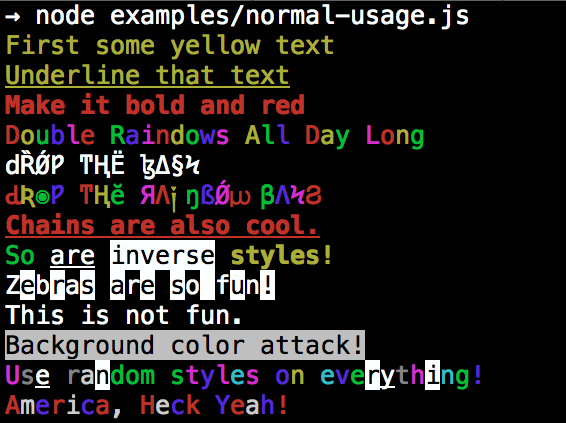 12 13## Installation 14 15 npm install colors 16 17## colors and styles! 18 19### text colors 20 21 - black 22 - red 23 - green 24 - yellow 25 - blue 26 - magenta 27 - cyan 28 - white 29 - gray 30 - grey 31 32### background colors 33 34 - bgBlack 35 - bgRed 36 - bgGreen 37 - bgYellow 38 - bgBlue 39 - bgMagenta 40 - bgCyan 41 - bgWhite 42 43### styles 44 45 - reset 46 - bold 47 - dim 48 - italic 49 - underline 50 - inverse 51 - hidden 52 - strikethrough 53 54### extras 55 56 - rainbow 57 - zebra 58 - america 59 - trap 60 - random 61 62 63## Usage 64 65By popular demand, `colors` now ships with two types of usages! 66 67The super nifty way 68 69```js 70var colors = require('colors'); 71 72console.log('hello'.green); // outputs green text 73console.log('i like cake and pies'.underline.red) // outputs red underlined text 74console.log('inverse the color'.inverse); // inverses the color 75console.log('OMG Rainbows!'.rainbow); // rainbow 76console.log('Run the trap'.trap); // Drops the bass 77 78``` 79 80or a slightly less nifty way which doesn't extend `String.prototype` 81 82```js 83var colors = require('colors/safe'); 84 85console.log(colors.green('hello')); // outputs green text 86console.log(colors.red.underline('i like cake and pies')) // outputs red underlined text 87console.log(colors.inverse('inverse the color')); // inverses the color 88console.log(colors.rainbow('OMG Rainbows!')); // rainbow 89console.log(colors.trap('Run the trap')); // Drops the bass 90 91``` 92 93I prefer the first way. Some people seem to be afraid of extending `String.prototype` and prefer the second way. 94 95If you are writing good code you will never have an issue with the first approach. If you really don't want to touch `String.prototype`, the second usage will not touch `String` native object. 96 97## Disabling Colors 98 99To disable colors you can pass the following arguments in the command line to your application: 100 101```bash 102node myapp.js --no-color 103``` 104 105## Console.log [string substitution](http://nodejs.org/docs/latest/api/console.html#console_console_log_data) 106 107```js 108var name = 'Marak'; 109console.log(colors.green('Hello %s'), name); 110// outputs -> 'Hello Marak' 111``` 112 113## Custom themes 114 115### Using standard API 116 117```js 118 119var colors = require('colors'); 120 121colors.setTheme({ 122 silly: 'rainbow', 123 input: 'grey', 124 verbose: 'cyan', 125 prompt: 'grey', 126 info: 'green', 127 data: 'grey', 128 help: 'cyan', 129 warn: 'yellow', 130 debug: 'blue', 131 error: 'red' 132}); 133 134// outputs red text 135console.log("this is an error".error); 136 137// outputs yellow text 138console.log("this is a warning".warn); 139``` 140 141### Using string safe API 142 143```js 144var colors = require('colors/safe'); 145 146// set single property 147var error = colors.red; 148error('this is red'); 149 150// set theme 151colors.setTheme({ 152 silly: 'rainbow', 153 input: 'grey', 154 verbose: 'cyan', 155 prompt: 'grey', 156 info: 'green', 157 data: 'grey', 158 help: 'cyan', 159 warn: 'yellow', 160 debug: 'blue', 161 error: 'red' 162}); 163 164// outputs red text 165console.log(colors.error("this is an error")); 166 167// outputs yellow text 168console.log(colors.warn("this is a warning")); 169 170``` 171 172### Combining Colors 173 174```javascript 175var colors = require('colors'); 176 177colors.setTheme({ 178 custom: ['red', 'underline'] 179}); 180 181console.log('test'.custom); 182``` 183 184*Protip: There is a secret undocumented style in `colors`. If you find the style you can summon him.* 185