README.md
1## fast_float number parsing library: 4x faster than strtod
2
3/badge.svg)
4/badge.svg)
5
6
7
8[](https://github.com/fastfloat/fast_float/actions/workflows/vs16-ci.yml)
9
10The fast_float library provides fast header-only implementations for the C++ from_chars
11functions for `float` and `double` types. These functions convert ASCII strings representing
12decimal values (e.g., `1.3e10`) into binary types. We provide exact rounding (including
13round to even). In our experience, these `fast_float` functions many times faster than comparable number-parsing functions from existing C++ standard libraries.
14
15Specifically, `fast_float` provides the following two functions with a C++17-like syntax (the library itself only requires C++11):
16
17```C++
18from_chars_result from_chars(const char* first, const char* last, float& value, ...);
19from_chars_result from_chars(const char* first, const char* last, double& value, ...);
20```
21
22The return type (`from_chars_result`) is defined as the struct:
23```C++
24struct from_chars_result {
25 const char* ptr;
26 std::errc ec;
27};
28```
29
30It parses the character sequence [first,last) for a number. It parses floating-point numbers expecting
31a locale-independent format equivalent to the C++17 from_chars function.
32The resulting floating-point value is the closest floating-point values (using either float or double),
33using the "round to even" convention for values that would otherwise fall right in-between two values.
34That is, we provide exact parsing according to the IEEE standard.
35
36
37Given a successful parse, the pointer (`ptr`) in the returned value is set to point right after the
38parsed number, and the `value` referenced is set to the parsed value. In case of error, the returned
39`ec` contains a representative error, otherwise the default (`std::errc()`) value is stored.
40
41The implementation does not throw and does not allocate memory (e.g., with `new` or `malloc`).
42
43It will parse infinity and nan values.
44
45Example:
46
47``` C++
48#include "fast_float/fast_float.h"
49#include <iostream>
50
51int main() {
52 const std::string input = "3.1416 xyz ";
53 double result;
54 auto answer = fast_float::from_chars(input.data(), input.data()+input.size(), result);
55 if(answer.ec != std::errc()) { std::cerr << "parsing failure\n"; return EXIT_FAILURE; }
56 std::cout << "parsed the number " << result << std::endl;
57 return EXIT_SUCCESS;
58}
59```
60
61
62Like the C++17 standard, the `fast_float::from_chars` functions take an optional last argument of
63the type `fast_float::chars_format`. It is a bitset value: we check whether
64`fmt & fast_float::chars_format::fixed` and `fmt & fast_float::chars_format::scientific` are set
65to determine whether we allow the fixed point and scientific notation respectively.
66The default is `fast_float::chars_format::general` which allows both `fixed` and `scientific`.
67
68The library seeks to follow the C++17 (see [20.19.3](http://eel.is/c++draft/charconv.from.chars).(7.1)) specification.
69* The `from_chars` function does not skip leading white-space characters.
70* [A leading `+` sign](https://en.cppreference.com/w/cpp/utility/from_chars) is forbidden.
71* It is generally impossible to represent a decimal value exactly as binary floating-point number (`float` and `double` types). We seek the nearest value. We round to an even mantissa when we are in-between two binary floating-point numbers.
72
73Furthermore, we have the following restrictions:
74* We only support `float` and `double` types at this time.
75* We only support the decimal format: we do not support hexadecimal strings.
76* For values that are either very large or very small (e.g., `1e9999`), we represent it using the infinity or negative infinity value.
77
78We support Visual Studio, macOS, Linux, freeBSD. We support big and little endian. We support 32-bit and 64-bit systems.
79
80
81
82## Using commas as decimal separator
83
84
85The C++ standard stipulate that `from_chars` has to be locale-independent. In
86particular, the decimal separator has to be the period (`.`). However,
87some users still want to use the `fast_float` library with in a locale-dependent
88manner. Using a separate function called `from_chars_advanced`, we allow the users
89to pass a `parse_options` instance which contains a custom decimal separator (e.g.,
90the comma). You may use it as follows.
91
92```C++
93#include "fast_float/fast_float.h"
94#include <iostream>
95
96int main() {
97 const std::string input = "3,1416 xyz ";
98 double result;
99 fast_float::parse_options options{fast_float::chars_format::general, ','};
100 auto answer = fast_float::from_chars_advanced(input.data(), input.data()+input.size(), result, options);
101 if((answer.ec != std::errc()) || ((result != 3.1416))) { std::cerr << "parsing failure\n"; return EXIT_FAILURE; }
102 std::cout << "parsed the number " << result << std::endl;
103 return EXIT_SUCCESS;
104}
105```
106
107
108## Reference
109
110- Daniel Lemire, [Number Parsing at a Gigabyte per Second](https://arxiv.org/abs/2101.11408), Software: Pratice and Experience 51 (8), 2021.
111
112## Other programming languages
113
114- [There is an R binding](https://github.com/eddelbuettel/rcppfastfloat) called `rcppfastfloat`.
115- [There is a Rust port of the fast_float library](https://github.com/aldanor/fast-float-rust/) called `fast-float-rust`.
116- [There is a Java port of the fast_float library](https://github.com/wrandelshofer/FastDoubleParser) called `FastDoubleParser`.
117- [There is a C# port of the fast_float library](https://github.com/CarlVerret/csFastFloat) called `csFastFloat`.
118
119
120## Relation With Other Work
121
122The fastfloat algorithm is part of the [LLVM standard libraries](https://github.com/llvm/llvm-project/commit/87c016078ad72c46505461e4ff8bfa04819fe7ba).
123
124The fast_float library provides a performance similar to that of the [fast_double_parser](https://github.com/lemire/fast_double_parser) library but using an updated algorithm reworked from the ground up, and while offering an API more in line with the expectations of C++ programmers. The fast_double_parser library is part of the [Microsoft LightGBM machine-learning framework](https://github.com/microsoft/LightGBM).
125
126## Users
127
128The fast_float library is used by [Apache Arrow](https://github.com/apache/arrow/pull/8494) where it multiplied the number parsing speed by two or three times. It is also used by [Yandex ClickHouse](https://github.com/ClickHouse/ClickHouse) and by [Google Jsonnet](https://github.com/google/jsonnet).
129
130
131## How fast is it?
132
133It can parse random floating-point numbers at a speed of 1 GB/s on some systems. We find that it is often twice as fast as the best available competitor, and many times faster than many standard-library implementations.
134
135<img src="http://lemire.me/blog/wp-content/uploads/2020/11/fastfloat_speed.png" width="400">
136
137```
138$ ./build/benchmarks/benchmark
139# parsing random integers in the range [0,1)
140volume = 2.09808 MB
141netlib : 271.18 MB/s (+/- 1.2 %) 12.93 Mfloat/s
142doubleconversion : 225.35 MB/s (+/- 1.2 %) 10.74 Mfloat/s
143strtod : 190.94 MB/s (+/- 1.6 %) 9.10 Mfloat/s
144abseil : 430.45 MB/s (+/- 2.2 %) 20.52 Mfloat/s
145fastfloat : 1042.38 MB/s (+/- 9.9 %) 49.68 Mfloat/s
146```
147
148See https://github.com/lemire/simple_fastfloat_benchmark for our benchmarking code.
149
150
151## Video
152
153[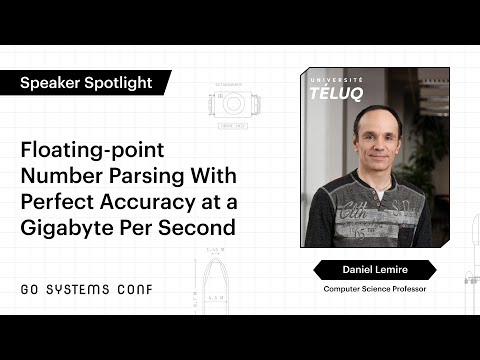](http://www.youtube.com/watch?v=AVXgvlMeIm4)<br />
154
155## Using as a CMake dependency
156
157This library is header-only by design. The CMake file provides the `fast_float` target
158which is merely a pointer to the `include` directory.
159
160If you drop the `fast_float` repository in your CMake project, you should be able to use
161it in this manner:
162
163```cmake
164add_subdirectory(fast_float)
165target_link_libraries(myprogram PUBLIC fast_float)
166```
167
168Or you may want to retrieve the dependency automatically if you have a sufficiently recent version of CMake (3.11 or better at least):
169
170```cmake
171FetchContent_Declare(
172 fast_float
173 GIT_REPOSITORY https://github.com/lemire/fast_float.git
174 GIT_TAG tags/v1.1.2
175 GIT_SHALLOW TRUE)
176
177FetchContent_MakeAvailable(fast_float)
178target_link_libraries(myprogram PUBLIC fast_float)
179
180```
181
182You should change the `GIT_TAG` line so that you recover the version you wish to use.
183
184## Using as single header
185
186The script `script/amalgamate.py` may be used to generate a single header
187version of the library if so desired.
188Just run the script from the root directory of this repository.
189You can customize the license type and output file if desired as described in
190the command line help.
191
192You may directly download automatically generated single-header files:
193
194https://github.com/fastfloat/fast_float/releases/download/v1.1.2/fast_float.h
195
196## Credit
197
198Though this work is inspired by many different people, this work benefited especially from exchanges with
199Michael Eisel, who motivated the original research with his key insights, and with Nigel Tao who provided
200invaluable feedback. Rémy Oudompheng first implemented a fast path we use in the case of long digits.
201
202The library includes code adapted from Google Wuffs (written by Nigel Tao) which was originally published
203under the Apache 2.0 license.
204
205## License
206
207<sup>
208Licensed under either of <a href="LICENSE-APACHE">Apache License, Version
2092.0</a> or <a href="LICENSE-MIT">MIT license</a> at your option.
210</sup>
211
212<br>
213
214<sub>
215Unless you explicitly state otherwise, any contribution intentionally submitted
216for inclusion in this repository by you, as defined in the Apache-2.0 license,
217shall be dual licensed as above, without any additional terms or conditions.
218</sub>
219